PDC10: Building a Blog with Microsoft "Unnamed Package of Web Love"
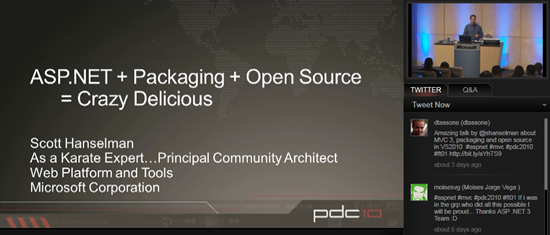
I did lots of stuff, met lots of people, did hours of "Live TV" on Channel 9 Live and presented a talk on behalf of the Web Platform and Tools team (WPT is IIS, Media Streaming, ASP.NET, everything web, etc) showcasing all the cool stuff we've been doing lately.
You can stream the talk with Silverlight Smooth Streaming as well as PIP (Picture in Picture) or you can download the talk in one of four formats. Note that for some weird reason there's 10 minutes of "we haven't started yet" dead video that you'll have to seek past. You should Right-Click Save As the video you want.
UPDATE: All these videos have been re-squished with better in-sync audio and a shorter lead-in.
- Low Bandwidth MP4 Video (about 120 megs)
- High Bandwidth MP4 Video (I dunno, it's big, and HD)
- Your Best Choice: Low Bandwidth WMV Video (about 258 megs)
- HighDef 720p WMV Video (about 800 megs)
If you noticed in my recent post introducing NuGet (was NuPack) I mentioned at the bottom that I was changing roles at Microsoft. I feel strongly that the Lego pieces we're building are the right size and that the Web Platform is moving in a direction that I want to influence more than I could while working in MSDN. I'll be working with the Razor, WebMatrix, IIS Express, SQL 4 CE+EF and ASP.NET WebForms and MVC teams building community on what I lovingly call "Unnamed Package of Web Thingies." Oh, and I'll also help name this stuff. Trust me, I'm voting against the usual names you've come to love us for. Anyway.
I gave this talk. A pile of folks were involved even though it's one random dude (me) on stage to make demos like this happen. PDC talks are also kind of "here's what we're thinking and what you could do in the future" type talks, so there was a LOT of daily builds and a lot of hacks/rough code that was put together for that talk. I wanted to share it with you so you can play with it and be involved in the conversation. The scaffolding stuff I showed was a proof of concept built on a prototype resting on a sample. That said, it's certainly a direction we want to head in.
In this post I'll show you how you can do the same demo yourself (mostly) with today's bits. I'll show you what'll work today with huge hacks and bug fixes, and how it'll be better tomorrow. I'm interested in your thoughts on how you'd like things to work tomorrow. I'm mostly interested in how you/us (the community) will build better stuff with these Lego, rather than just taking this stuff and callign it done. Cause it ain't done.
Building a Blog with Microsoft "Unnamed Package of Web Love"
Of course, watch the video to see how I do it and follow along, but here's what you'll need first.'
Remember, you asked for it, so this is the crazy version. The some alternate future version would more likely be "Get VS2010 and one other thing" and it'll just work™.
- Visual Studio 2010 and ASP.NET MVC 3.
- Get whatever version is current. As of today, that's ASP.NET MVC 3 Beta. An RC will be out soon. Doesn't matter, most of this will work. I'll point out weird stuff as we go.
- NuGet Alpha Build from 10-26
- Get this alpha and install the VSIX by double clicking it. It's alpha, so expect you WILL have to uninstall it at some point. No it won't mess up your system.
- In Tools | Options | Package Manager, make sure you're pointing to http://go.microsoft.com/fwlink/?LinkId=204820. That's currently a redirect to http://feed.nuget.org/ctp2/odata/v1/. At some point in the future it'll be automatic and easy.
- Worst case scenario and you want to uninstall it, Run VS2010 as Admin, to go Tools | Extension Manager and Uninstall it.
Again, disclaimer, this isn't a tutorial for new folks to learn ASP.NET MVC. This is a behind the curtains transparent "here's what I did for this proof of concept demo and I'm interested in your feedback as fellow geeks." Read carefully.
Here's the demo.
New Project
Go File | Note the new View Engine drop down. Perhaps Spark, Nhaml, etc will get in this dropdown at some point. We'll choose Razor. Run the default template. Note that it's using Visual Web Development Server since that's what's built in.
Alpha/Beta Software Hack Warning: Now, let's pretend this is a "Choose Your Own Adventure" book. You can skip this part, or you can do this hack.
I was using daily build stuff that enabled IIS Express to be installed on its own outside of WebMatrix (our small, free Web Development IDE). You'll get this ability in the future and it'll be built into Visual Studio in some way similar to my demo. For now, you can either.
1. Don't sweat IIS Express and move on. Continue to use Visual Web Developer. Things will work fine.
or
2. Try to hack IIS Express in. Here's how, assuming you have WebMatrix installed.
Open Notepad, paste in:
SET ExecPath=%ProgramFiles(x86)%
IF "%ExecPath%" == "" SET ExecPath = "%ProgramFiles%
"%ExecPath%\Microsoft WebMatrix\iisexpress.exe" /path:"C:\PATHTOMY\MvcApplication3" /port:12345
Save this file as "thisisahack.bat" somewhere and run it. You'll start and stop this on your own, remembering that it'll be built in someday. Go into your ASP.NET MVC applications Properties, click Web, the under "Use Custom Web Server" click that radio button and paste in http://localhost:12345 as the URL.
Get a package with NuGet...I need a DB
Now, I need a database, so I'll use the new SQLCE with the Entity Framework. From inside Visual Studio, show the Package Manager Console. Two ways, either Ctrl-W then Ctrl-Z or View | Other Windows | Package Manager Console.
Alpha/Beta Software Warning: From inside the NuGet console, you'll install a bunch of beta libraries with this command:
Install-Package SQLCE.EntityFramework
After this you'll get:
Successfully installed 'SQLCE.EntityFramework 4.0.8435.1'
Successfully added 'SQLCE 4.0.8435.1' to MvcApplication3
Successfully added 'EFCTP4 1.0' to MvcApplication3
Successfully added 'WebActivator 1.0.0.0' to MvcApplication3
Successfully added 'SQLCE.EntityFramework 4.0.8435.1' to MvcApplication3
Note the stuff that got added to your references and the new AppStart_SQLCEEntityFramework.cs that showed up. This is using the EFCTP4 (Entity Framework Magic Unicorn) I've blogged about before as well as SQL Compact Edition 4, a tiny xcopyable file based SQL Server. BOTH of these are not released products. All these came down off the live NuGet feed and will most likely be updated as they can be.
Create Models
Make a new file in the Models folder called something like BlogContext.cs. Put this in it and confirm you have the right namespaces. Something like this:
using System;
using System.Collections.Generic;
using System.Data.Entity;
namespace MvcApplication3.Models //yours will be different
{
public class Post
{
public int ID { get; set; }
public string Title { get; set; }
public string Text { get; set; }
public DateTime PublishDate { get; set; }
public virtual ICollection<Comment> Comments { get; set; }
}
public class Comment
{
public int ID { get; set; }
public int PostID { get; set; }
public string Text { get; set; }
public string Author { get; set; }
}
public class BlogContext : DbContext
{
public DbSet<Post> Posts { get; set; }
public DbSet<Comment> Comments { get; set; }
}
}
This should compile at this point. Now I need a controller for this blog of ours. Instead of making in with the Add Controller dialog, I'll go back to the Package Manager Console.
Create Controller for Blog
Install a new package...this time some totally horrible experimental scaffolding that started with David Ebbo, squeezed by David Fowler, then ruined by myself.
Alpha/Beta Software Hack Warning: There's blood on all our hands for this one. Feel free to dig into this package, if you can handle the evil, and look at some of the ideas being explored.
Install-Package MvcScaffold
They made me rename it. You'll be getting version 0.2. In this case, we're still using NuGet, except in an unusual way. We're not adding libraries to the project, rather we're extending the PowerShell environment with new functions.
Make sure you compile at least once before you run this! The PowerShell scripts are going to wander around in the project looking for types and they won't exist as CLR types if they haven't been compiled. One of the added method was Scaffold-Controller:
Scaffold-Controller -m Post -c BlogContext
This will creates the controller against the context and also create CRUD views.
Note that you have a new folder created in your project called CodeTemplates. I've talked about T4 (Text Template Transformation Toolkit) before as well as in a recorded talk in the Netherlands called "ASP.NET MVC 2: Ninja Black Belt Tips" while wearing a horrible sweater.
This CodeTemplates folder is a folder that you'll actually find deep inside the beast around: C:\Program Files (x86)\Microsoft Visual Studio 10.0\Common7\IDE\VWDExpress\ItemTemplates\CSharp\Web\MVC 3 or something similar. As I show in my Ninja video, you can copy those templates out and override them simply by putting them local to your project. Once they are local, they can be edited to do whatever we like.
Hack Warning: I'm adding these to the project and we're using them in a slightly unconventional way. T4 templates - as the tooling is today - assume they will run before your build or when a file or your project is dirty/changed. Adding all these T4 templates at once causes Visual Studio to freak out and pop up "run this template" dialogs for every template. Also, once they are added they have a Custom Tool associated with them. I have done one thing and I propose another. First, I changed the extension of these files from .tt to .t4 to prevent that dialog from coming up. Changing the extension makes Visual Studio think nothing of them. Now, I propose that 3rd party T4 tools should know about this extension and enable syntax highlighting for these files. An extension of .TT runs as usual and the .T4 extension means no tooling runs. This way .T4 files can be includes, or in my example/demo here, just files that are "along for the ride and used occasionally."
Back to the Scaffolding. The Scaffold-Controller method used the ControllerWithContext.t4 template to generate the Controller for me directly against the Entity Framework CTP4 DbContext. The Views were generated from the Razor views you see in the CSHTML folder.
Compile and it should run.
Run application and visit /Post
At this point, I actually goofed up but I didn't want to change the scaffolding as it was 3am when I realized it. I should have pluralized things and make a Posts (plural) Controller rather than a PostController. I'd like to have the Entity Framework team give me a public API for "PluralizeThisThing()" or something. There's no reason for me to know about Octopus:Octopi.
Anyway, hit http://localhost:12345/Post and you'll see:
Hit Create New, add a Post and it'll show up in the list. Yay.
Now, if you get this YSOD (Yellow Screen of Death) with SQL Compact Edition 4 CTP that declares "File already exists. Try using a different database name" here's the deal. Remember what I said about Hacks and Bugs?
Hack Bug Work Around Warning: There's a bug in the SQL Compact Edition 4 CTP (CTP==Beta) that gets confused about if the database exists or not. If you hit this, for now, hit F5.
Choose Your Own Adventure Time. You can:
1. Hit F5 and it'll just work©
2. Change the Database Initializer (you can do that, since things are more pluggable in Microsoft land now) and introduce this hack. Yes, it'll be fixed before it comes out.
Go into your AppStart_SQLCEEntityFramework.cs file and the DefaultConnectionFactory line to this:
Database.DefaultConnectionFactory = new SqlCeConnectionFactory(
"System.Data.SqlServerCe.4.0",
HostingEnvironment.MapPath("~/App_Data/"),"");
At this point, you should be able to:
- See listing of posts (although none there to begin with)
- Create New Post, now it shows up in list.
- Click details to see the post.
At this point I have CRUD, I have a "just in time created SQL Compact Edition DB" in my App_Data folder. The code in my controller is going against the Entity Framework DbContext directly.
Trick: In my talk I showed Tooling that will let you open the SDF (SQL Database File) and make tables, edit things, etc. You don't have that because it's coming. There ARE tools out there though, if you really really want to see inside the SQL Compact Edition 4 database, like http://sqlcetoolbox.codeplex.com. But you didn't hear it from me.
Now, do note that the CRUD (Create, Read, Update, Delete) views were generated off the Model. Our blog posts have Title and Text and both are a single line. A blog's contents needs multiline text boxes (textareas) so there's two things we could do. If the template generated TextBoxFor() or TextAreaFor() based on some metadata, we could add [DataType(DataType.MultilineText)] to the Text field and regenerate the views passing in -f for "Force." Try typing a dash, the hitting tab. You get Intellisense in this PowerShell, also.
But, since I've changed my templates to use EditorFor, I just need to add [DataType(DataType.MultilineText)] to the Text property of my Database and run my app again. The right Editor (TextBox or TextArea) is chosen at runtime, not compile- or scaffold-time.
Make Views pretty
The main list of posts is a table, and is not really nice for a blog. Let's look at the Razor syntax and make it the site nicer. Also, I'll change the Site.css to use the one from our designer. You can get the Site.css from here.
With Razor, if I want to spin through some posts, I could delete the generated table and add this to my Post\Index.cshtml file:
<div class="blog-posts">Or even just this. Notice how it knows that the ! isn't part of the expression?
@foreach (var item in Model) {
@item.Text
}
</div>
<div>@item.Text!</div>
I'll just spin through the posts, set some CSS and layout my page.
<div class="blog-posts">And here's better code for Post\Details.cshtml:
@foreach (var item in Model) {
<ul class="blog-post">
<li class="blog-title">@Html.ActionLink(item.Title, "Details", new { id=item.ID })</li>
<li class="blog-content">@MvcHtmlString.Create(item.Text)</li>
<li class="meta">Publish Date</li>
<li class="meta">@String.Format("{0:g}", item.PublishDate)</li>
<li class="meta">
@Html.ActionLink("Edit", "Edit", new { id=item.ID }) |
@Html.ActionLink("Details", "Details", new { id=item.ID }) |
@Html.ActionLink("Delete", "Delete", new { id=item.ID })
</li>
</ul>
<hr />
}
</div>
<h2 class="blog-title">@Model.Title</h2>
<ul class="blog-post">
<li class="blog-content">@MvcHtmlString.Create(Model.Text)</li>
<li class="meta">Publish Date</li>
<li class="meta">@String.Format("{0:g}", Model.PublishDate)</li>
</ul>
Beta Note: Those places where we have MvcHtmlString.Create will probably turn into something easier like Html.Raw(). All Html strings are encoded unless you explicitly say otherwise.
You can hit refresh in browser after each change in Razor to incrementally see your page changing.
Better models with validation attributes
We don't have any data validation so I'll add add [Required] for everything, and StringLength[50] for Title, and whatever else you like. If I run my app now, I've got data validation.
However, this validation doesn't work until I click Create. It's server-side. Useful, but not taking advantage of JavaScript.
Beta Note: This is all stuff that could be set in the default template, meaning we wouldn't have to do any of this by default. Maybe just comment out some JS.
Let's turn on client-side validation in web.config. Note that it's using unobtrusive JavaScript, which is a fancy way of saying "no generated javascritp arrays." It's a good thing and it makes for tidier HTML:
<add key="ClientValidationEnabled" value="true" />
<add key="UnobtrusiveJavaScriptEnabled" value="true" />
Add these scripts to Create.cshtml and Edit.cshtml:
<script src="/Scripts/jquery-1.4.1.js" type="text/javascript"></script>
<script src="/Scripts/jquery.validate.js" type="text/javascript"></script>
<script src="/Scripts/jquery.validate.unobtrusive.js" type="text/javascript"></script>
Now, if we re-run the application and try to create a post, validation works great on the client side. This is all new in ASP.NET MVC 3.
Add Comments
Our blog needs comments. Let's not scaffold comments, just add a controller without CRUD using Right Click | Add Controller.
public class CommentController : Controller
{
public ActionResult Create(Comment c)
{
BlogContext db = new BlogContext();
Post post = db.Posts.Single(p => p.ID == c.PostID);
post.Comments.Add(c);
db.SaveChanges();
return RedirectToAction("Details", "Post", new { ID = c.PostID });
}
}
Then we'll modify the Details.cshtml view and have it include a Form to POST to the Comments controller.
Beta Note: You won't have syntax highlighting or intellisense for Razor yet. Soon.
@using(Html.BeginForm("Create", "Comment")) {
<div id="comments">
<input type="hidden" name="PostID" value="@Model.ID" />
<label for="Author">Email:</label> <input type="text" name="Author"/>
<label for="Text">Comment:</label> <textarea name="Text" rows="5" cols="40"></textarea>
<input type="submit" value="Add Comment"/>
</div>
}
And then add a foreach within Details to list the comments, if there are any.
<h2 class="comments-title">Comments</h2>
<ul class="comments">
@foreach(var comment in Model.Comments) {
<li class="group">
@comment.Author says: @comment.Text
</li>
}
</ul>
This is lovely, and will work if you run it, but I didn't use the Repository Pattern before when accessing the database. Let's rescaffold the PostController, except this time with a basic Repository.
Change to a Repository Pattern
It sure would be nicer if I could do something like this:
IPostRepository repo;
public CommentController(IPostRepository r)
{
repo = r;
}
public ActionResult Create(Comment c)
{
Post p = repo.GetById(c.PostID);
p.Comments.Add(c);
repo.Save();
return RedirectToAction("Details", "Post", new { ID = c.PostID });
}
I can make a Repository class in \Models with this:
Scaffold-Repository -m Post -c BlogContext
Let's change Post to use a Repository...make sure to include Force and NoViews. Force forces overwriting and -NoViews doesn't do Views.
Scaffold-Controller -m Post -c Blog -r Post -f -NoViews
Then I'll check out the code for the PostController and add a little sort since this is a blog.
return View(this.repository.GetAllPosts().OrderByDescending(p => p.PublishDate));
But, if I run this, notice that I never actually MADE an IPostRepository. This is a good time for IoC (Inversion of Control)
Add IOC
We will add not just Ninject (a popular IoC container) but an MVC3 specific package that will help us wire things up.
Install-Package Ninject.MVC3
Now, look at AppStart_NinjectMVC3.cs. Add this:
kernel.Bind<IPostRepository>().To<PostRepository>();
...and the app will run again as Ninject is using the new DependencyResolver in ASP.NET MVC 3.
// Tell ASP.NET MVC 3 to use our Ninject DI Container
DependencyResolver.SetResolver(new NinjectServiceLocator(kernel));
Bring in a Helper for Gravatars
I'd like to add some Gravatars to my comments as they look boring.
Again, back to the Package Management Console:
Install-Package microsoft-web-helpers
This is an interesting package, however, as it's filled with Razor Helpers that are great for using in ASP.NET Web Pages (that's the simpler stuff that you see in WebMatrix). These are effectively WebMatrix Helpers. But, since they are Razor and this is all ASP.NET, they work nicely.
@Gravatar.GetHtml(comment.Author)
Then, I just F5 in the browser...and:
Finally in my demo I added Windows Live Writer support using Charles Cook's XML-RPC library, much like I did in this recent blog post. That's more involved so I'll talk about that other day.
Conclusion
Things are coming together nicely with Visual Studio 2010 plus Microsoft "Unnamed Package of Web Love." There's more to come and more needed, like Database Migrations.
In the short term, the MvcScaffold package is a 0.2 prototype. I think it could be the beginnings of an interesting generic scaffolding that could be extended by the community (you could share CodeTemplate folders) and extended to do Tests, BDD, whatever. We are interested in your thoughts about how T4-based dynamic-using templates could be used along with Razor, SQLCE4, EF Code-First as well as the pile of awesome Open Source Libraries getting added to the http://www.nuget.org project daily.
Your thoughts, Dear Reader?
Related Links
- Introducing NuGet Package Management for .NET - Another piece of the Web Stack
- Simple Code First with Entity Framework 4 - Magic Unicorn Feature CTP 4
- "Razor" Page Syntax
- SQL 4 Compact Edition
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
public class BlogContext : DbContext
{
public DbSet<Post> Posts { get; set; }
public DbSet<Comment> Comments { get; set; }
}
to
public class BlogContext : DbContext
{
public DbSet<Post> Posts { get; set; }
}
Where you can only add a comment through the Posts property, after all a comment has to have a post.
Basically be able to export object graphs via only their root objects.
Oh yeah, and send them over to Silverlight and edit, add, delete then send them back a ChangeSet and have EF update properly.
Can you please forward this feedback to relevant person.
IMO the key to success is the quality of the package install into your app. In other words don't ever impose on the user anything other than typing the install package command to have it fully working in their app. It just works.
To accomplish this I would suggest the NuGet team focuses on creating a rich manifest and helper methods that can make this job easier for the package creators.
I also love the command line approach. We don't need no stinkin UI :)
Great job!
The following error occurred while loading the extended type data file: Microsoft.PowerShell.Core, C:\Windows\SysWOW64\WindowsPowerShell\v1.0\types.ps1xml(2971) : Error in type "System.Security.AccessControl.ObjectSecurity": Exception: Cannot convert the "Microsoft.PowerShell.Commands.SecurityDescriptorCommandsBase" value of type "System.String" to type "System.Type".
Congrats on the new position. Related question: When can we expect IIS Express to drop standalone? I have a real need for this at work and am anxious to get hold of it for the team. Since the announcement in July, news has been dark.
And thanks for posting that link to the css. Please have your team designers produce some more site.css files. I can't design worth a flop and I am not happy with many of the styles on the MvcContrib Template Gallery.
Thanks,
Terrence
Updating solved it for me. A little birdie (let's call him "Flott Panselpenn") helped me solve this.
The following error occurred while loading the extended type data file: Microsoft.PowerShell.Core, C:\Windows\SysWOW64\WindowsPowerShell\v1.0\types.ps1xml(2971) : Error in type "System.Security.AccessControl.ObjectSecurity": Exception: Cannot convert the "Microsoft.PowerShell.Commands.SecurityDescriptorCommandsBase" value of type "System.String" to type "System.Type".
In the video scott shows the Database in the Server Explorer. I cant do this
yet.
PM> Scaffold-Controller -m Post -c BlogContext
New-Object : Cannot find type [ScaffoldingFramework.DynamicViewModel]: make sure the assembly containing this type is loaded.
At C:\svn\testmvc\packages\MvcScaffold.0.2\tools\init.ps1:37 char:35
+ $dynamicModel = New-Object <<<< ScaffoldingFramework.DynamicViewModel
+ CategoryInfo : InvalidType: (:) [New-Object], PSArgumentException
+ FullyQualifiedErrorId : TypeNotFound,Microsoft.PowerShell.Commands.NewObjectCommand
Cannot index into a null array.
At C:\svn\testmvc\packages\MvcScaffold.0.2\tools\init.ps1:40 char:23
+ $dynamicModel[ <<<< "Namespace"] = $project.Properties.Item("DefaultNamespace").Value
+ CategoryInfo : InvalidOperation: (Namespace:String) [], RuntimeException
+ FullyQualifiedErrorId : NullArray
Cannot index into a null array.
At C:\svn\testmvc\packages\MvcScaffold.0.2\tools\init.ps1:47 char:23
+ $dynamicModel[ <<<< "FrameworkVersion"] = $frameworkName.Version
+ CategoryInfo : InvalidOperation: (FrameworkVersion:String) [], RuntimeException
+ FullyQualifiedErrorId : NullArray
Now I get this:
PM> Scaffold-Controller -m Post -c BlogContext
Added file 'Controllers\PostController.cs'
Unknown type 'Post'
At C:\svn\testmvc\packages\MvcScaffold.0.2\tools\init.ps1:279 char:26
+ throw <<<< "Unknown type '$ModelType'"
+ CategoryInfo : OperationStopped: (Unknown type 'Post':String) [], RuntimeException
+ FullyQualifiedErrorId : Unknown type 'Post'
But the postcontroller gets created and builds.
Thank you for the very interesting talk Scott :-)
Seriously Scott, you are a presenter I really admire - would be great to see you 'on the other side' one day - the water's warm, go on jump in - you know you want to one day!
How about replace the t4 templates with razor templates?
Description: An error occurred during the compilation of a resource required to service this request. Please review the following specific error details and modify your source code appropriately.
Compiler Error Message: CS1973: 'System.Web.Mvc.HtmlHelper<dynamic>' has no applicable method named 'ActionLink' but appears to have an extension method by that name. Extension methods cannot be dynamically dispatched. Consider casting the dynamic arguments or calling the extension method without the extension method syntax.
Source Error:
Line 13:
Line 14: <ul class="blog-post">
Line 15: <li class="blog-title">@Html.ActionLink(item.Title, "Details", new { id=item.ID })</li>
Line 16: <li class="blog-content">@MvcHtmlString.Create(item.Text)</li>
Line 17: <li class="meta">Publish Date</li>
PM> Install-Package mvcscaffold
Successfully installed 'MvcScaffold 0.2'
Install-Package : Cannot bind argument to parameter 'Path' because it is null.
At line:1 char:16
+ Install-Package <<<< mvcscaffold
+ CategoryInfo : NotSpecified: (:) [Install-Package], ParameterBindingValidatio
nException
+ FullyQualifiedErrorId : NuGet.VisualStudio.Cmdlets.InstallPackageCmdlet
Then, when attempting to use a command from the package:
Scaffold-Controller -m Post -c BlogContext
The term 'Scaffold-Controller' is not recognized as the name of a cmdlet, function, script fi
le, or operable program. Check the spelling of the name, or if a path was included, verify th
at the path is correct and try again.
At line:1 char:20
+ Scaffold-Controller <<<< -m Post -c BlogContext
+ CategoryInfo : ObjectNotFound: (Scaffold-Controller:String) [], CommandNotFou
ndException
+ FullyQualifiedErrorId : CommandNotFoundException
robert ivanc - Did you fix your issue? Are you in an MVC2 or MVC3 application?
Anton - Yes, that was lame and now it's fixed by the PDC team. All the videos were re-rendered I'm told.
Brian and Geetha - Let me ask the team.
Did you try building before running the scaffolding commands? You need to build so that the powershell scripts can find the types.
Can you file a bug on codeplex with the repro steps? http://nuget.codeplex.com/
I've filed an item at http://nuget.codeplex.com/workitem/383, thanks. I've also included a couple more details on my environment in that post.
I have some misunderstanding:
If I'm trying to do something like this
@if (!string.IsNullOrEmpty(comment.Author)) { @Gravatar.GetHtml(comment.Author) }
The gravatar rendered and it's greate!
BUT
Then I tried to do it like this:
@if (!string.IsNullOrEmpty(comment.Author)) { Gravatar.GetHtml(comment.Author); }
Gravatars doesn't shown... Text Gravatar.GetHtml(comment.Author); doesn't printed too...
The question is WHY? Where is the logic?
PS. Awesome show! I like your style.
The issue is that without the "@", you're just running the GetHtml method and doing nothing with the return value (which is the HTML to be rendered). When you put "@" in front of it, that tells Razor that you want to call the method AND render the result to the page.
On the video, when Scott changed his model, DB was updated on the fly. When I'm changing the model, I always get the exception, which says me that I have to update/recreate DB...
Maybe I should install something special? :)
if (!Context.Database.ModelMatchesDatabase() && Context.Database.Exists())
Context.Database.Delete();
Context.Database.CreateIfNotExists();
Context.Database.EnsureInitialized();
Be aware though that you will, of course, loose any data you've added to the DB already.
(Hopefully ModelMatchesDatabase() contains efficient code to check this, but I always say that you should always assume that code you haven't written yourself is as stupid as possible, just in case.)
Database.SetInitializer<YourContextImplementation>(new RecreateDatabaseIfModelChanges<YourContextImplementation>());
Last solution working pretty well (for now) :)
Why didn't I thought of it...
Next I was able to run the scaffold against a Entity Design done through the EntityDesigner (so DB First if you may), using Regular SQL Server and not the SQLCE Entity Framework
I did run into the following error in the Scaffold command and the View generator saying;
Compiling transformation: The type or namespace name 'Data' does not exist in the namespace 'System' (are you missing an assembly reference?)
This may happen even with said assembly namespaces are included in the project.
Solution for me was to close Visual Studio/ Restart as Administrator. Now I've set the shortcut to run as Admin always. This was inspite of my login being an admin account on Windows 7 (x64). Hope this helps.
I need help for this!
Scaffold-Controller -ModelType Post -ContextType BlogContext -ProjectName Bloggy
In China, there is an old saying, meet both the affinity,
For our fate, cheers.
First meeting. I first introduced to our company's products,
We mainly produce
fuel dispenser, china fuel dispenser, Petrol dispenser, fuel dispenser pump, fuel dispenser manufacturer, fuel dispenser parts, fuel dispenser nozzle, oil dispenser, High flow fuel dispenser, Heavy Duty Fuel Dispenser.
If you are interested you can see here
my site http://www.sankichina.com
Disturb you. Please forgive me.
Freshly updated Visual Studio 2010 sp1beta and latest Asp.Net MVC 3 RC2.
Everything compiles nicley before i try to scaffold the controller and views.
The controller gets added but it fails to create the views.
I tried with:
PM> Scaffold-Controller -m Post -c BlogContext
Added file 'Controllers\PostController.cs'
Unknown type 'Post'
At C:\users\andreas\documents\visual studio 2010\Projects\packages\MvcScaffold.0.2\tools\init.ps1:279 char:26
+ throw <<<< "Unknown type '$ModelType'"
+ CategoryInfo : OperationStopped: (Unknown type 'Post':String) [], RuntimeException
+ FullyQualifiedErrorId : Unknown type 'Post'
And also as suggested by mosman.myopenid.com:
PM> Scaffold-Controller -ModelType Post -ContextType BlogContext -ProjectName MvcApplication3
PostController.cs already exists! Skipping...
Unknown type 'Post'
At C:\users\andreas\documents\visual studio 2010\Projects\packages\MvcScaffold.0.2\tools\init.ps1:279 char:26
+ throw <<<< "Unknown type '$ModelType'"
+ CategoryInfo : OperationStopped: (Unknown type 'Post':String) [], RuntimeException
+ FullyQualifiedErrorId : Unknown type 'Post'
Anyone who solved this allready or has ideas about it?
Starting from an empty MVC3 RC2 web project, you need to:
[In Solution Explorer]
right-click on the MVC project
Add > | Add ASP.NET Folder | App_Data
I'm having some trouble with the package manager console. When I supply command List-Package, it gives me the output that "No packages installed". However I can search the list of packages from "Add Library Package Reference" dialog. Instead of, I'd like to prefer the command-line way. Can you guide me how to get that list in PM console?
Thanks,
Pravesh
PM> List-Package
No packages installed.
PM> list-package -installed
Get-Package : A parameter cannot be found that matches parameter name 'installed'.
At line:1 char:24
+ list-package -installed <<<<
+ CategoryInfo : InvalidArgument: (:) [Get-Package], ParameterBindingException
+ FullyQualifiedErrorId : NamedParameterNotFound,NuGet.VisualStudio.Cmdlets.GetPackageCmdlet
FYI: I'm using
NuGet v1.0.10128.89
VS 2010 SP1 Beta
An FYI, I have MvcScaffold 0.3 installed. I was using it to build the controller, repository, and views. The repository didn't build until I ran Scaffold-Repository by itself. Here's the output.
PM> scaffold-controller -ModelType User -ContextType DiscoveryContext -Repository User -f -NoViews
Added file 'Controllers\UserController.cs'
PM> scaffold-controller -m User -c DiscoveryContext -r User -f -NoViews
Added file 'Controllers\UserController.cs'
PM> Scaffold-Repository -m User -c DiscoveryContext
Added file 'Models\UserRepository.cs'
In response to Pravesh Soni:
I had to use "List-Package -Remote" to get a list of the online packages. You could try that.
Hope it helps!
/Robert
Great presentation, as were your previous ones on MVC. One thing that you sort of gloss over when you talk about using SQL Server Ce, is that the default membership provider code that is added by the project template uses SQL Server Express. This means that, even if you NuGet the SQLCe bits, as soon as you try to register a user, you will create the membership MDF and use SQL Server Express for login stuff.
Unfortunately, this means that the site is no longer XCopy deployable. (or msdeploy-able to a machine without SQL Server Express installed).
Is it possible to make the membership code use SQLCe as a provider instead of SQLExpress? And, if so, how to go about it? My goog... ..errm .... bing-fu apparently isn't up to the task of finding it.
be well
-h-
Anyway, this project on CodePlex does just what I was looking for.
be well
Comments are closed.
--larsw