The whole of WordPress compiled to .NET Core and a NuGet Package with PeachPie
Why? Because it's awesome. Sometimes a project comes along that is impossibly ambitious and it works. I've blogged a little about Peachpie, the open source PHP compiler that runs PHP under .NET Core. It's a project hosted at https://www.peachpie.io.
But...why? Here's why:
- Performance: compiled code is fast and also optimized by the .NET Just-in-Time Compiler for your actual system. Additionally, the .NET performance profiler may be used to resolve bottlenecks.
- C# Extensibility: plugin functionality can be implemented in a separate C# project and/or PHP plugins may use .NET libraries.
- Sourceless distribution: after the compilation, most of the source files are not needed.
- Power of .NET: Peachpie allows the compiled WordPress clone to run in a .NET JIT'ted, secure and manageable environment, updated through windows update.
- No need to install PHP: Peachpie is a modern compiler platform and runtime distributed as a dependency to your .NET project. It is downloaded automatically on demand as a NuGet package or it can be even deployed standalone together with the compiled application as its library dependency.
A year ago you could very happily run Wordpress (a very NON-trivial PHP application, to be clear) under .NET Core using Peachpie. You would compile your PHP into an assembly and then do something like this in your Startup.cs:
public void Configure(IApplicationBuilder app) { app.UseSession(); app.UsePhp(new PhpRequestOptions(scriptAssemblyName: "peachweb")); app.UseDefaultFiles(); app.UseStaticFiles(); }
And that's awesome. However, I noticed something on their GitHub recently, specifically under https://github.com/iolevel/wpdotnet-sdk. It says:
The solution compiles all of WordPress into a .NET assembly and additionally provides C# wrappers for utilization of compiled sources.
Whoa. Drink that in. The project consists of several parts:
wordpress
contains sources of WordPress that are compiled into a single .NET Core assembly (wordpress.dll
). Together with its content files it is packed into a NuGet packagePeachPied.WordPress
. The project additionally contains the "must-use" pluginpeachpie-api.php
which exposes the WordPress API to .NET.PeachPied.WordPress.Sdk
defines abstraction layer providing .NET interfaces over PHP WordPress instance. The interface is implemented and provided bypeachpie-api.php
.PeachPied.WordPress.AspNetCore
is an ASP.NET Core request handler that configures the ASP.NET pipeline to pass requests to compiledWordPress
scripts. The configuration includes response caching, short URL mapping, various .NET enhancements and the settings of WordPress database.app
project is the executable demo ASP.NET Core web server making use of compiledWordPress
.
They compiled the whole of WordPress into a NuGet Package.
YES.
- The compiled website runs on .NET Core
- You're using ASP.NET Core request handling and you can extend WordPress with C# plugins and themes
Seriously. Go get the .NET Core SDK version 2.1.301 over at https://dot.net and clone their repository locally from https://github.com/iolevel/wpdotnet-sdk.
Make sure you have a copy of mySQL running. I get one started FAST with Docker using this command:
docker run --name=mysql1 -p 3306:3306 -e MYSQL_ROOT_PASSWORD=password -e MYSQL_DATABASE=wordpress mysql --default-authentication-plugin=mysql_native_password
Then just "dotnet build" at the root of the project, then go into the app folder and "dotnet run." It will show up on localhost:5004.
NOTE: I needed to include the default authentication method to prevent the generic Wordpress "Cannot establish database connection." I also added the MYSQL_DATABASE environment variable so I could avoid logging initially using the mysql client and creating the database manually with "CREATE DATABASE wordpress."
Look at that. I have my mySQL in one terminal listening on 3306, and ASP.NET Core 2.1 running on port 5004 hosting freaking WordPress compiled into a single NuGet package.
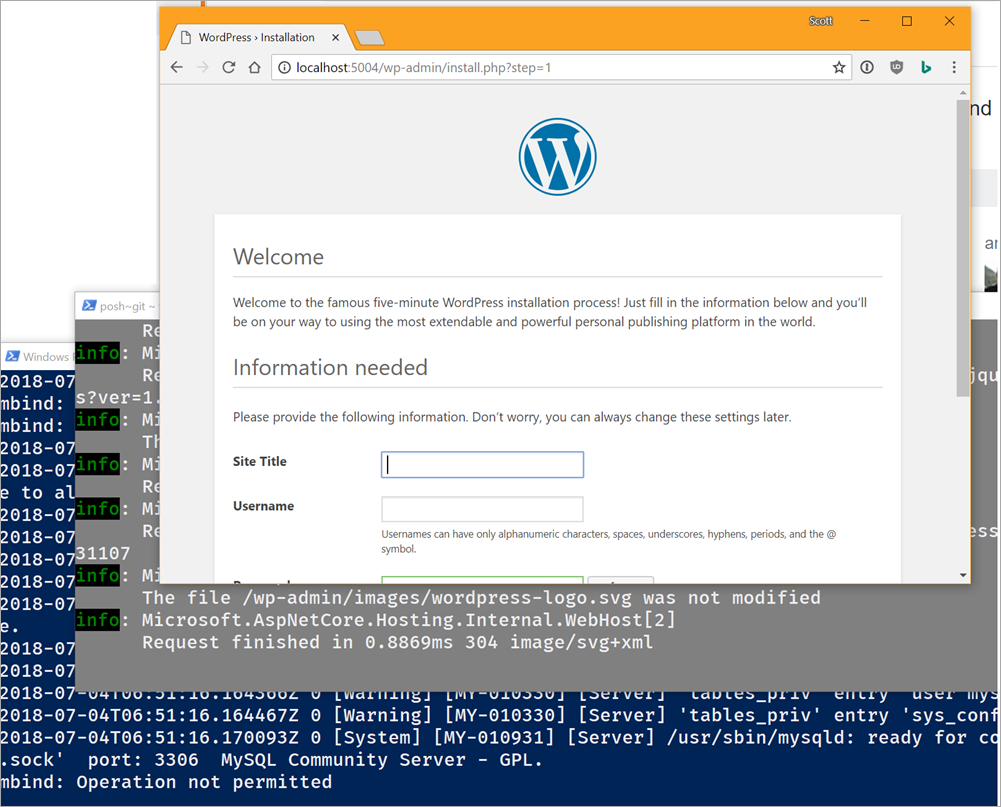
Here's my bin folder:
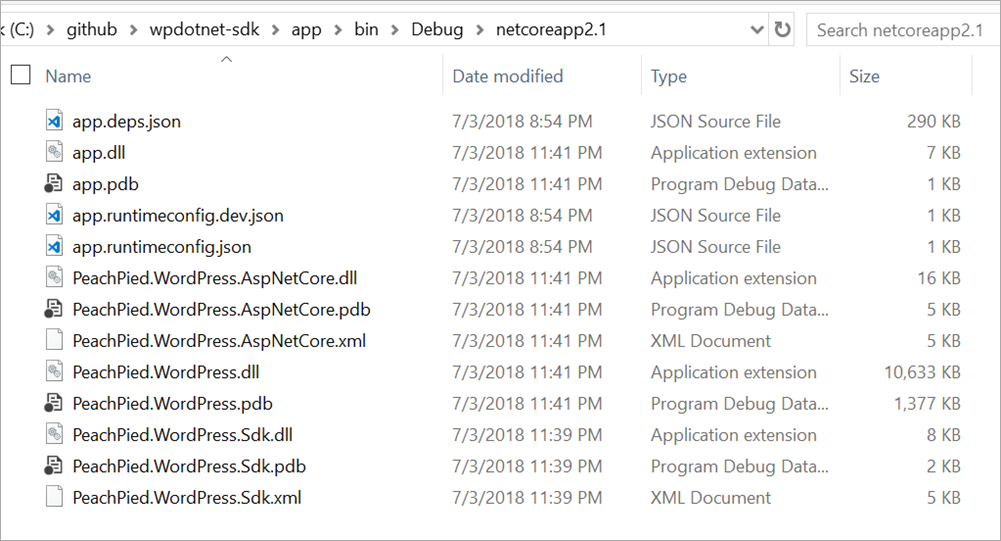
There's no PHP files which is a nice security bonus - not only are you running from the one assembly but there's no text files for any rogue plugins to modify or corrupt.
Here's the ASP.NET Core 2.1 app that hosts it, in full:
using System.IO; using Microsoft.AspNetCore; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using PeachPied.WordPress.AspNetCore; namespace peachserver { class Program { static void Main(string[] args) { // make sure cwd is not app\ but its parent: if (Path.GetFileName(Directory.GetCurrentDirectory()) == "app") { Directory.SetCurrentDirectory(Path.GetDirectoryName(Directory.GetCurrentDirectory())); } // var host = WebHost.CreateDefaultBuilder(args) .UseStartup<Startup>() .UseUrls("http://*:5004/") .Build(); host.Run(); } } class Startup { public void Configure(IApplicationBuilder app, IHostingEnvironment env, IConfiguration configuration) { // settings: var wpconfig = new WordPressConfig(); configuration .GetSection("WordPress") .Bind(wpconfig); // if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseWordPress(wpconfig); app.UseDefaultFiles(); } } }
I think the app.UseWordPress() is such a nice touch. ;)
I often get emails from .NET developers asking what blog engine they should consider. Today, I think you should look closely at Peachpie and strongly consider running WordPress under .NET Core. It's a wonderful open source project that brings two fantastic ecosystems together! I'm looking forward to exploring this project more and I'd encourage you to check it out and get involved with Peachpie.
Sponsor: Check out dotMemory Unit, a free unit testing framework for fighting all kinds of memory issues in your code. Extend your unit testing with the functionality of a memory profiler!
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
Any advice will be appreciated. Thanks
TM
There is a lightweight demo project https://github.com/iolevel/peachpie-wordpress that uses that compiled WordPress - you can add your custom plugins and themes and just hit F5 in VSCode.
Use of the WP Dashboard to modify your sources is not allowed (and not gonna work), however; I think use of a regular code editor like VSCode gives you much better experience.
Most of the hacked WordPress sites I've seen are due to weak password on admin account. By weak, I mean crazy weak. Think 1234.
But then again, the people who will run WordPress through PeachPie are bound to have better password practices than that.
Gotcha. There are definitely some limitations to the CMS, albeit to be fair we haven't actually done many code optimizations, so we still have a bunch of room for improvement.
@David:
Plugins - yes, when you add a new plugin, you will have to recompile, but with the new SDK you will only be compiling the actual plugin, while the main WP app remains an already compiled NuGet reference.
Updates - actually one of the big advantages we can offer is that the constant WP updates can be performed seamlessly in the background from our feed of already compiled and CI-tested builds, so you won't have to worry about updates. Plus with .NET you don't really care about most of the security fixes, which is the bulk of why WP updates so often.
And I have huge respect for Mr. Hanselman.
At first, I thought, "oh, this is interesting".
I mean no disrespect to the obviously talented people that pulled this off.
But IMO, suggesting that .NET programmers should consider this, instead of one of the many .NET CMS or blog engine projects is just plain bad advice.
Comments are closed.
And... Who are the crazy guys behind that?