How to set up Kubernetes on Windows 10 with Docker for Windows and run ASP.NET Core
Docker for Windows is really coming along nicely. They have both a Stable and Edge channel and the Edge (beta, experimental) one just included a lovely new feature - Kubernetes support. Per their docs, Kubernetes is only available in Docker for Windows 18.02 CE Edge. They set most everything up nicely and put Kubectl into your path and setup a context. If you use kubectl for other things - like your own Raspberry Pi Kubernetes Cluster, then you'll need to be aware of switching contexts. Same thing applies if you have one in the cloud, like the Kubernetes Cluster I made in Azure AKS.
Got Docker for Windows? If you have not yet installed Docker for Windows, see Install Docker for Windows for an explanation of stable and edge channels, system requirements, and download/install information.
It's easy to get started, just click "Enable Kubernetes" and Docker for Windows will download and start the images you need. I clicked "show system containers" because I like to see what's hidden from me, but you decide for yourself. Do be aware - there's a TON.
By default, you won't get the Kubernetes Dashboard - of which I'm a fan - so you may want to install that. If you follow the default instructions (and you're a noob like me) then you'll likely end up with a Dashboard that is pretty locked down. It can be somewhat frustrating to get access to your own development dashboard, so I use the alternative (read: totally insecure) dashboard, like this:
C:\> kubectl apply -f https://raw.githubusercontent.com/kubernetes/dashboard/master/src/deploy/alternative/kubernetes-dashboard.yaml
I also like charts and graphs so I added these as well:
C:\> kubectl create -f https://raw.githubusercontent.com/kubernetes/heapster/master/deploy/kube-config/influxdb/influxdb.yaml C:\> kubectl create -f https://raw.githubusercontent.com/kubernetes/heapster/master/deploy/kube-config/influxdb/heapster.yaml C:\> kubectl create -f https://raw.githubusercontent.com/kubernetes/heapster/master/deploy/kube-config/influxdb/grafana.yaml
I can access the dashboard by default by running "kubectl proxy" then visiting this http://localhost:8001/ui and I'll get redirected to the dashboard:
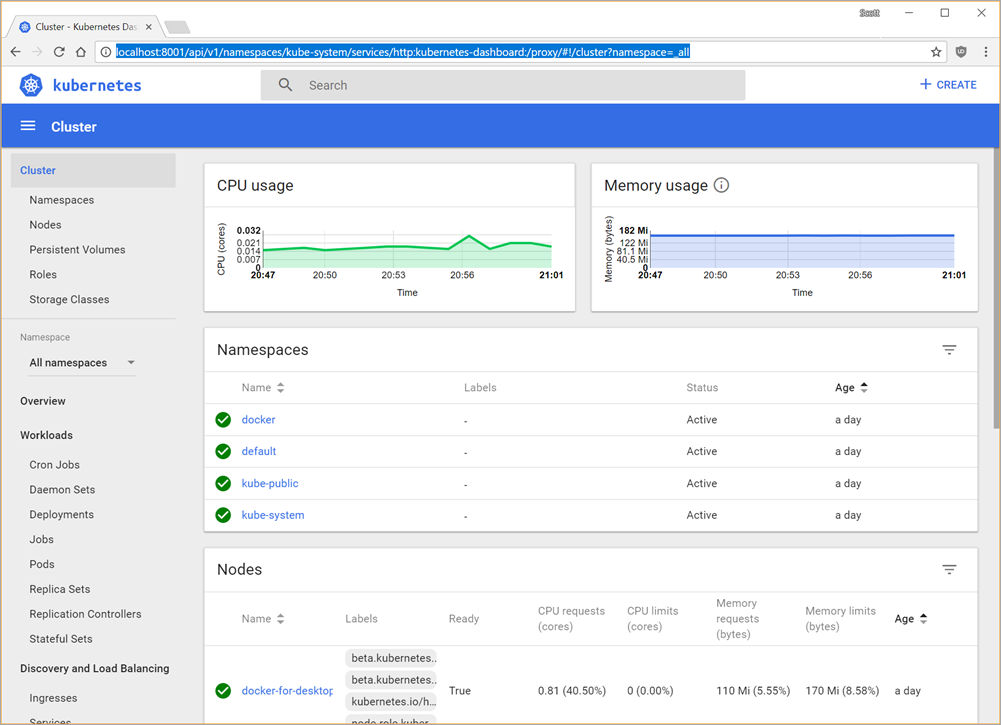
Now I can run through all the cool Kubernetes tutorials like the Guestbook Kubernetes Sample Application from the convenience of my Windows 10 machine. (I'm running a SurfaceBook 2 on the current non-Beta Windows 10.)
There are a lot of nice samples on running .NET Core and ASP.NET Core apps with Docker up at https://github.com/dotnet/dotnet-docker-samples/
I made a quick ASP.NET Core app called kubeaspnetapp:
C:\Users\scott\Desktop>dotnet new razor -o kubeaspnetapp The template "ASP.NET Core Web App" was created successfully. ...snip... Restore succeeded.
Then added a two-stage build DockerFile that looks like this:
FROM microsoft/aspnetcore-build:2.0 AS build-env WORKDIR /app # copy csproj and restore as distinct layers COPY *.csproj ./ RUN dotnet restore # copy everything else and build COPY . ./ RUN dotnet publish -c Release -o out # build runtime image FROM microsoft/aspnetcore:2.0 WORKDIR /app COPY --from=build-env /app/out . ENTRYPOINT ["dotnet", "kubeaspnetapp.dll"]
And built and tagged the image with:
C:\Users\scott\Desktop\kubeaspnetapp>docker build -t kubeaspnetapp:v1 .
Then I create a quick Deployment that manages a Pod that runs the Container:
C:\Users\scott\Desktop\kubeaspnetapp>kubectl run kubeaspnetapp --image=kubeaspnetapp:v1 --port=80 deployment "kubeaspnetapp" created
Now I'll expose it to the "outside." Again, this is usually done with .yaml files but it's a good learning exercise and it's all local.
C:\Users\scott\Desktop\kubeaspnetapp>kubectl get deployments NAME DESIRED CURRENT UP-TO-DATE AVAILABLE AGE kubeaspnetapp 1 1 1 1 1m C:\Users\scott\Desktop\kubeaspnetapp>kubectl get pods NAME READY STATUS RESTARTS AGE kubeaspnetapp-778f6d49bd-rct59 1/1 Running 0 1m C:\Users\scott\Desktop\kubeaspnetapp> C:\Users\scott\Desktop\kubeaspnetapp> C:\Users\scott\Desktop\kubeaspnetapp>kubectl expose deployment kubeaspnetapp --type=NodePort service "kubeaspnetapp" exposed C:\Users\scott\Desktop\kubeaspnetapp>kubectl get services NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE kubeaspnetapp LoadBalancer 10.98.234.67 <pending> 80:31756/TCP 5s kubernetes ClusterIP 10.96.0.1 <none> 443/TCP 1d
Then I'll hit http://127.0.0.1:31756 in my browser...note how that port is brokering to the internal port 80 where the app listens...and there's my ASP.NET Core app running locally on Kubernetes, set up with Docker for Windows. Nice.
Here's me getting the startup logs from that pod:
C:\Users\scott\>kubectl get pods NAME READY STATUS RESTARTS AGE kubeaspnetapp-7fd7f7ffb9-8gnzd 1/1 Running 0 6m C:\Users\scott\Dropbox\k8s for pi\aspnetcoreapp>kubectl logs kubeaspnetapp-7fd7f7ffb9-8gnzd Hosting environment: Production Content root path: /app Now listening on: http://[::]:80 Application started. Press Ctrl+C to shut down.
Pretty cool. As all the tooling and things across Windows, Docker, Kubernetes, Visual Studio (all flavors) continues to get better and better, I can only imagine this experience will get better and better. I look forward to a time when I can freely mix containers from different OSs and easily push them all en masse to Azure.
Sponsor: Get the latest JetBrains Rider for debugging third-party .NET code, Smart Step Into, more debugger improvements, C# Interactive, new project wizard, and formatting code in columns.
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
kubectl apply -f https://raw.githubusercontent.com/kubernetes/dashboard/master/src/deploy/alternative/kubernetes-dashboard.yaml
serviceaccount "kubernetes-dashboard" configured
role "kubernetes-dashboard-minimal" configured
rolebinding "kubernetes-dashboard-minimal" configured
service "kubernetes-dashboard" configured
The Deployment "kubernetes-dashboard" is invalid: spec.template.metadata.labels: Invalid value: map[string]string{"k8s-app":"kubernetes-dashboard"}: `selector` does not match template `labels`
kubectl proxythat starts the UI proxy server on 127.0.0.1:8001 I was able to see the Kubernetes UI
I am unable to deploy the local image. However, when I pushed the image to docker hub, deployment got successful.
Comments are closed.