Xamarin.Forms - Write Once, Run Everywhere, AND Be Native?
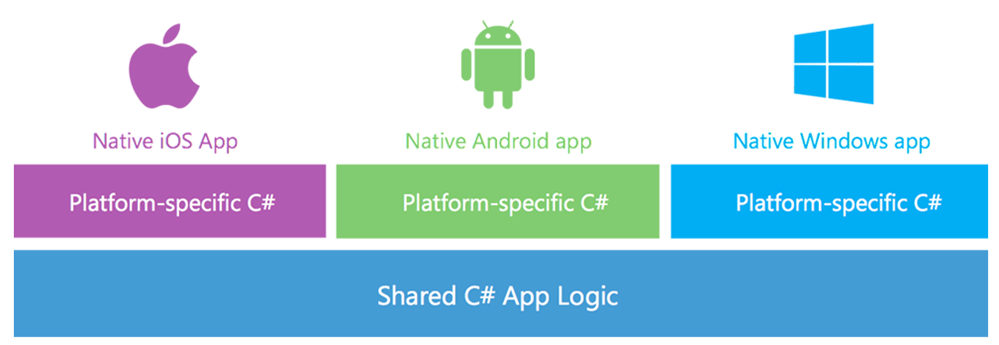
I worked in Java for a number of years at Nike, writing an order management application that would run on four platforms. We used to joke that we'd "write once, debug everywhere." Now, this was the early days of Java, but the thing was, every form and control was 'owner drawn.' That meant that a button looked the same everywhere because it wasn't a real button as far as the operating system was concerned. It was a picture of a button. We used to use Spy++ and different Windows inspector programs to explore our applications and they could never see a Java program's controls. This meant that the app pretty much worked everywhere, but the app always LOOKED like a Java App. They didn't integrated with the underlying platform.
With MVVM (Model, View, View-Model) patterns, and techniques like the Universal apps work on Windows Phone 8.1 and Windows 8.1, code sharing can get up into the high 90% for some kinds of apps. However, even for simple apps you've still got to create a custom native view for each platform. This is desirable in many cases, but for some app it's just boring, error prone, and tedious.
Xamarin announced Xamarin.Forms today which (in my words) effectively abstracts away native controls to a higher conceptual level. This, to my old eyes, is very similar to the way I wrote code in Java back in the day - it was all done in a fluent code-behind with layouts and flows. You create a control tree.
"Xamarin.Forms is a new library that enables you to build native UIs for iOS, Android and Windows Phone from a single, shared C# codebase. It provides more than 40 cross-platform controls and layouts which are mapped to native controls at runtime, which means that your user interfaces are fully native."
What's interesting about this, to me, is that these "control/concepts" (my term) are coded at a high level but rendered as their native counterparts. So a "tab" in my code is expressed in its most specific and native counterpart on the mobile device, rather than as a generic tab control as in my Java example. Let's see an example.
My buddy from Xamarin, James Montemagno, a fellow Chipotle lover, put together the ultimate cross-platform Hanselman application in a caffeinated late night hack to illustrate a few points for me. This little app is written in C# and runs natively on Windows Phone, Android, and iOS. It aggregates my blog and my tweets.
Here is the menu that switches between views:
And the code that creates it. I've simplified a little for clarity, but the idea is all MVVM:
public HomeMasterView(HomeViewModel viewModel) { this.Icon = "slideout.png"; BindingContext = viewModel; var layout = new StackLayout { Spacing = 0 }; var label = new ContentView { Padding = new Thickness(10, 36, 0, 5), BackgroundColor = Color.Transparent, Content = new Label { Text = "MENU", Font = Font.SystemFontOfSize (NamedSize.Medium) } }; layout.Children.Add(label); var listView = new ListView (); var cell = new DataTemplate(typeof(ListImageCell)); cell.SetBinding (TextCell.TextProperty, HomeViewModel.TitlePropertyName); cell.SetBinding (ImageCell.ImageSourceProperty, "Icon"); listView.ItemTemplate = cell; listView.ItemsSource = viewModel.MenuItems;
//SNIP
listView.SelectedItem = viewModel.MenuItems[0]; layout.Children.Add(listView); Content = layout; }
Note a few things here. See the ListImageCell? He's subclassed ImageCell, which is a TextCell with an Image, and setup data binding for the text and the icon. There's recognition that every platform will have text and an icon, but the resources will be different on each. That's why the blog and Twitter icons are unique to each platform. The concepts are shared and the implementation is native and looks native.
That's the UI side, on the logic side all the code that loads the RSS feed and Tweets is shared across all three platforms. It can use async and await for non-blocking I/O and in the Twitter example, it uses LinqToTwitter as a PCL (Portable Class Library) which is cool. For RSS parsing, it's using Linq to XML.
private async Task ExecuteLoadItemsCommand() { if (IsBusy) return; IsBusy = true; try{ var httpClient = new HttpClient(); var feed = "http://feeds.hanselman.com/ScottHanselman"; var responseString = await httpClient.GetStringAsync(feed); FeedItems.Clear(); var items = await ParseFeed(responseString); foreach (var item in items) { FeedItems.Add(item); } } catch (Exception ex) { var page = new ContentPage(); var result = page.DisplayAlert ("Error", "Unable to load blog.", "OK", null); } IsBusy = false; }
And ParseFeed:
private async Task<List<FeedItem>> ParseFeed(string rss) { return await Task.Run(() => { var xdoc = XDocument.Parse(rss); var id = 0; return (from item in xdoc.Descendants("item") select new FeedItem { Title = (string)item.Element("title"), Description = (string)item.Element("description"), Link = (string)item.Element("link"), PublishDate = (string)item.Element("pubDate"), Category = (string)item.Element("category"), Id = id++ }).ToList(); }); }
Again, all shared. When it comes time to output the data in a List on Windows Phone, Android, and iPhone, it looks awesome (read: native) on every platform without having to actually do anything platform specific. The controls look native because they are native. Xamarin.Forms controls are a wrapper on native controls, they aren't new controls themselves.
Here's BlogView. Things like ActivityIndicator are from Xamarin.Forms, and it expresses itself as a native control.
public BlogView () { BindingContext = new BlogFeedViewModel (); var refresh = new ToolbarItem { Command = ViewModel.LoadItemsCommand, Icon = "refresh.png", Name = "refresh", Priority = 0 }; ToolbarItems.Add (refresh); var stack = new StackLayout { Orientation = StackOrientation.Vertical, Padding = new Thickness(0, 8, 0, 8) }; var activity = new ActivityIndicator { Color = Helpers.Color.DarkBlue.ToFormsColor(), IsEnabled = true }; activity.SetBinding (ActivityIndicator.IsVisibleProperty, "IsBusy"); activity.SetBinding (ActivityIndicator.IsRunningProperty, "IsBusy"); stack.Children.Add (activity); var listView = new ListView (); listView.ItemsSource = ViewModel.FeedItems; var cell = new DataTemplate(typeof(ListTextCell)); cell.SetBinding (TextCell.TextProperty, "Title"); cell.SetBinding (TextCell.DetailProperty, "PublishDate"); cell.SetValue(TextCell.StyleProperty, TextCellStyle.Vertical); listView.ItemTapped += (sender, args) => { if(listView.SelectedItem == null) return; this.Navigation.PushAsync(new BlogDetailsView(listView.SelectedItem as FeedItem)); listView.SelectedItem = null; }; listView.ItemTemplate = cell; stack.Children.Add (listView); Content = stack; }
Xamarin Forms is a very clever and one might say, elegant, solution to the Write Once, Run Anywhere, AND Don't Suck problem. What's nice about this is that you can care about the underlying platform when you want to, and ignore it when you don't. A solution that HIDES the native platform isn't native then, is it? That'd be a lowest common denominator solution. This appears to be hiding the tedious and repetitive bits of cross-platform multi-device programming.
There's more on Xamarin and Xamarin Forms at http://xamarin.com/forms and sample code here. Check out the code for the Hanselman App(s) at https://github.com/jamesmontemagno/Hanselman.Forms.
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
note: I know the build issue is currently an issue b/c of apple not allowing it on pcs and not xamarins doing
Cheers,
Jason Wyglendowski
It's great that you can test the whole suit or have a free edition, but let's be honest: the app size for the free package is too small, even for semi-serious apps. You can't even use HttpClient and/or Json.Net without reaching the max. size of the app :(
At the moment I'm pretty sure that Xamarin is targeting businesses and doesn't bother that much about individual (hobby) developers or enthusiasts. It'd be great if Xamarin could offer free licenses for OSS projects or allow people to get a license for a monthly subscription of 10$ (or something like that), like Epic Games did with their Unreal Engine and Crytek with their CryEngine.
Considering the productivity gain that you get for cross platform development, 40 bucks a month isn't expensive, that's half an hour of work.
Hell, the Visual Studio support costs more than Visual Studio itself.. and even more so since it's a yearly subscription. Makes me sad.
Now that Xamarin has abstracted the UI, the value of their product just increased; there's no way we're going to see a price drop. They're sitting on a gold mine, why should they give it away?
Our only real hope is that Microsoft acquires Xamarin and rolls that into MSDN without increasing the cost of MSDN.
As for the cost of the Enterprise licenses? Yes, they're steep. They're for ENTERPRISE. You DON'T need Visual Studio. I've been using Xamarin Studio (formerly MonoDevelop) to write apps since 2011. Visual Studio is a nice-to-have, not a necessity. If you're a startup, you can HANDILY accomplish your goals with Xamarin Studio (which is FREE).
The indy plan is also pretty cruddy - not even VS support - which is what their major value comes from. The fact that the free edition can't even support the most basic of http apps without hitting the limits is really, really frustrating (for fun I tried to make an app front-end for a freeware tournament thing I'm working on: I hit the limit before I even had it receiving all the data).
I get they want to make money and clearly the product is absolutely worth a fair penny but yeah - there's no way it's even kind of justifiable without a sensible expectation of making money.
Unfortunately, that's also par for the course with a lot of UI Control Libraries. Mad props to MahApp for their efforts there.
Can I continue to use Xamarin when my subscription expires?
Yes. Your Xamarin license is perpetual. If you choose not to renew your subscription, you will no longer have access to new releases and support, and we will be very sad.
Even if I went with the Indie version as others have mentioned Xmarain Studio works very well for $299 it is very reasonably priced.
The tooling keeps getting better each year and makes mobile native cross platform mobile development fun and easy. The email support and forums are excellent and it just keeps getting better.
Also the annual pricing is to keep current with new releases, which is a very common practice.
Do we just consider Xamarin in cases where we have a connected application with a nice backend and just need a native shell?
As for Xamarin.Forms... It's a nice idea for marketing, but if you want your app to stand out I don't think you should use it. The iOS designer looks fantastic though.
If you're not happy then go do the following:
- Learn Objective C
- Learn Java
- (C# knowledge assumed)
- Learn the different UI methodologies for each of the above
- Setup the different development environments for each of the above
- Setup the different build environments for each of the above
So you can waste your time doing all of the above or pony up the money. Choice is up to you. How do you value your time? Let's do the math:
- $3,000 = 3 business licenses so you can target all 3 platforms
- 30 hours = Time to recoup cost @ $100ph
So you only need to find 30 hours of billable time per year and you've paid for it. Seems like a no brainer to me.
And that is why Xamarin tools are so expensive. The only way they will be less expensive is if they had comparable competition.
Let's not forget, though, that the Xamarin folks have been essentially on this project for more than a decade. They've paid their dues, they've put years of work in, and they've had their bad times (when Novell jettisoned them). If anybody deserves to make some money, it's them.
Cheers,
Jason Wyglendowski
You almost had me for a minute, but I've seen this song and dance before. I'm not going to fall for it again.
For a professional developer, who has determined to enter the mobile app market, it's still a small cost that should be easily recouped if they have a serious business model. That's a topic for another day though! :)
With Xamarin.Forms library and its shared user interface controls and layouts you would build out the views in the code behind or you can build it with and XAML markup. In this example I did it all from the code behind. We have tons of samples and docs on http://www.xamarin.com/forums
I'm not taking a position on Xamarin's pricing (as it looks like an uber sweet product). But I did want to share this anecdote about how big company's work regarding custom tools.
Be well.
- using Xamarin studio after using VS is just heart breaking. It does a reasonable job but when you KNOW Xamarin works on VS if you've got an extra $1000 lying around it does make you weep.
- Xamarin studio (prior to 3) was horrible at Nuget and PCL's. I tried to do the 'smart' thing and develop my app in VS for WP8, then just open up the solution in Xamarin studio to do the UI for Android and iOS. Xamarin studio failed so hard with the PCL that it became a whitewash and I ended up abandoning the whole idea and just shadow linking the files instead. I watched a few videos on MVVM-Cross which had my hyped to get going but notice how all the videos use the VS edition, that's for a reason folks. Try to find someone that's got MVVM-Cross working well from Xamarin Studio
- Using a Mac as a purely Windows user is more challenging that you might think. Why is my screen now a clock and a calculator, why can't I maximize a Window to take up the full screen without losing the ability to quickly switch apps, why is the file picker so much harder to use. why does ctrl+c not work (It's all apple key). etc.. Not starting a Mac vs PC debate here but just recognize that the two OS's operate differently. Using VS here and just linking the Mac to do a build would save me hours of swearing at the computer at least.
- With Typescript getting released and a template for Cordova working from within Visual Studio it seems that I'll happily trade 10-20% performance for my app, for 80-90% happier me. If I was doing some blockbuster, barnstorming app I'd think differently. But for the niche apps that I write it's just not a factor.
All of the above could be solved by just letting the $299 work with VS and finding some other way to extract the higher price from enterprises.
Full credit to Xamarin though I love that they are gung-ho in their support for F#. Are you listening Microsoft, I remember being told that F# was a first class language, try telling that to my WP8 apps
Take an app on multiple platforms (say 3). Take the cost and time of implementation of those 3 platforms that you will be building seperately in silos (Objective C, Java and C#). If it's one person that is doing that, great, but could be 3 different resources you need to be paying for aswell. This is for building the apps and ofcourse long term maintenance. Also there are all the logistical costs of making that all work. Now take the cost of building an app once with a shareable core, with native coded UIs in C#. Subtract the two, and well Xamarin justifies it's price. People rarely consider the cost of time and effort, just see a $299 or $999 cost. That license cost is covered extremely quickly by developer hourly rate costs. Also I do find mostly complaints about the cost of the Visual Studio version of Xamarin is by people that do not have a legal license for Visual Studio itself anyways.
Points on MVVMCross. I use MvvmCross in both Visual Studio and Xamarin Studio. I'm not sure why the need for tutorials on all platforms, as they are exactly the same implementation in either IDE. It's MVVM and C#, IDE has nothing to do with it. MVVMCross is great. PCL libraries work just fine. And there are more and more PCL compatible libraries being added.
Points on having to have a Mac. I'm a Windows person, and don't really like a Mac myself. However you can comfortably get away with rarely using your Mac. A Mac-mini is very cheap (and this is from somebody in South Africa with a horrible Rand dollar exchange rate) and if you use the build host you can spend your time mostly in windows and rarely have to worry about the Mac. This is even better now that there are designers in Visual Studio for iOS in Xamarin 3. OSX also runs happily in a VM so you don't have to have a physical Mac and every thing works from there including provisioning to a Device. The need for a Mac though is not Xamarin's need, it's Apple's need. And if you are building an iOS app in Objective C, you need a Mac anyways.
On Cordova. Also went on a Cordova mission a while back, and I can safely say every one of those efforts is in the bin as the result was not good at all. Performance is terrible, and rendering issues were even worse across the myriad of devices all with different versions of browser engines. To enable usable experiences I found it had to be tailored for each platform anyways which defeated the purpose of the exercise. And it's lovely building an app on a platform, to discover another platform has none of those plugins.
What I have now is a large library of reusable code (for client and server code). Xamarin enables me to quickly take that code, reuse it and build apps fast. In the end doing that I don't notice the price and it justifies itself easily. However if you are building only 1 free app ever to put in an app store, then yes it's expensive. But that was going to be "expensive" any way you look at it.
If we are coding on Windows, we will be using VS...period... VS is the platinum standard for IDEs (IMHO) and anyone that has used it and than moved to another IDE (Eclipse, CodeBlock, NetBeans, MonoDevelop, Xamarin, vi/gdb, emacs/gdb, etc...) will be a sad camper in many ways... especially when it comes to quality of the IDE and the debugging support, even our in-house Xcode fanboys wish they had VS for debugging. (disclaimer: I am a vi/shell guy doing WinRemoting on OS-X for Linux & Windows but when on Windows I relish my coding time in VS)
Long story short, $1000 (x2) entry point for the VS plugin for each Window's dev, tester, DevOp, build eng, etc.. (make sure that you understand each 'platform' part of the licensing is another $1000 for Biz.). Roll in our OS-X/Xcode users and you are talking more licenses for Xamarin.Mac for porting our Windows desktop C# front-ends to native OS-X and they still need iOS/Andriod licenses also.
Since most devs work on apps for iOS and Android plus at least one desktop OS you have to almost double out the licensing ("Per platform, per developer" model). Now we were looking at a $100k+ quote assuming we do not buy the 'same' level of support that we have for VS, otherwise is it was the $190k quote and we still do not really have the same level of support and we would not even be using the MonoDevelop based IDE except to modify the project file (99% of the time; VS, Xamarin VS plugin, Xcode, Vi, Emacs, & Xamarin's build would be used, not the IDE) and each mobile platform's UI is coded to that platform's UI strengths, a lowest common denominator (LCD) UI approach does not work for product marketing or our customers (we tried with one app a while back and lost marketshare in that area), shared C# logic 'under' the UI on 5 platforms, sure... shared C# 'net/cloud services on Azure/AWS, fine... but no LCD UIs on desktop or mobile. Maybe for some quick prototyping, sure, but the licensing did not change to allow us to do that and thus it is still $190k/yr when we already have $500k just in VS and other code tooling licensing and are cranking 5 versions of each apps out the door (well not all apps are on all OSs) .... management just stared at us at the end of that meeting and we all though another 'Red Wedding' was going to happen as the ROI was not there for our business (maybe the other commentaries can get away with one $300/license for the Android app, but that does not work in our full dev team environment....) :-/
For us, we would love to either see Xamarin folded into Visual Studio Ultimate... hint hint... or a major change to the current licensing model...
I would love to use VS on the indie license though :).
C++ means native development and the real tool for Windows for building native applications is Visual C++.
I am very surprised to see that C# is native... Some folks will now believe that... Is it a new marketing directive ?
If the goal is to write ios apps using vs and C# I don't want to have a mac involved. Call me stubborn I don't care ;)
I understand this is a valuable and relatively inexpensive tool for serious developers. But it doesn't seem like there's a budget-friendly option for hobbyists and those wanting to try and see if they'd even be interested in becoming serious developers.
Personally I think the "Business Features" and the email support are plenty to justify the extra cost for a business license. Throw in cloud builds at that price point and you'll make it even more of an obvious value for that target market. But the $299 enthusiast level really needs to include VS support.
"Prime Components" represent a few components/libraries on our component store.
We created a little FAQ on our forums: http://forums.xamarin.com/discussion/17241
I get the pricing concerns, and I think a grand would be fair if it covered all platforms. Still, it's just too much to justify if you're a hobbyist trying to build your skill set or do something fun. Would love to do a companion app to one of my sites, like CoasterBuzz or something, but I don't do enough ad revenue to cover the cost.
Recently I decided to try Ionic Framework for a new iOS/Android app and was blown away with how nice of a dev experience it is. I was able to build a simple production-ready app in just a few hours worth of work, and it was even more fun than Xamarin! Yes you need to know Angular, and you'll eventually need to learn more about PhoneGap/Cordova which it's built on top of, but the out-of-the-box experience was a real productivity blast. A nice side benefit is that it's completely free.
I'll continue to use Xamarin at work since they pay for it (but due to the cost only some devs get iOS licenses and other devs get Android licenses so I don't get the multi-platform experience I'd like), but for any side projects and personal projects I'm now totally sold on the Ionic/PhoneGap approach.
This is what's wrong with the software industry. This is why we can't have nice things.
More at http://ambroselittle.svbtle.com/how-much-is-your-productivity-worth
http://blogs.msdn.com/b/msgulfcommunity/archive/2013/03/16/compile-in-the-cloud-with-wp8.aspx
...and w/ .NET Native (in preview), Windows Store apps will be native soon too:
http://blogs.msdn.com/b/dotnet/archive/2014/04/02/announcing-net-native-preview.aspx
With regards to form, i guess its not all or nothing, you can still opt in to create parts of your app with that and other parts using the native uikit for the platform?
For that cost, it should include a mac in cloud "like" service to eliminate the local onsite mac dependency.
This is the pricing model I think would make many of us happier:
One time fee's, not yearly. (pay to upgrade major revisions)
$99 no VS (one platform)
$199 no VS bring your own mac (all platforms)
$299 VS support bring your own mac (one platform)
$399 VS support bring your own mac (all platforms)
$499 VS support mac in cloud (one platform) with 3 months in cloud
$599 VS support mac in cloud (all platforms) with 3 months in cloud
After 3 months... ($50 a month for mac in cloud, or $499 per year) Could add that to any of the bring your own mac versions.
I won't be able to build my skill set by building some sample apps before I really decide if I can use it for my real world use cases.
Won't buy.
As a lone, moonlighting, indie developer, supporting just one platform and keeping the app's in-store rating high sounds like plenty of work, at least until the code base and feature set stabilize. Focusing on one platform at a time, allows me to refine the product without having to test it over and over. What sounds nice about Xam.Forms, is that when I am ready to support another platform, even less code will need to be ported/rewritten.
It's a great tool, a group of people put a lot of work into it (it shows) and I want it to have a long and happy future. I was happy using Delphi for about 15 years and I want to be using Xamarin and C# for a long time to come. I was giving Borland/Code Gear/Embarcadero about $1000 a year to keep that 'up to date' and to be honest in recent years they'd ship something riddled with bugs then instead of fixing them just ship a new version 12 months later. So far, Xamarin have been really quick to get stuff out, and get stuff fixed (I've had first hand experience of their informal support in the forums and it was good. I bet the paid-for support at Enterprise level is awesome).
If it's your day job, it's just part of what you have to pay for your tools. Even as a self-employed one-person business (around $1000 a year coming right out of my own pocket for Delphi), it's just the cost of keeping your tools up to date.
The annual pricing shouldn't be an issue really - the mobile platforms are very dynamic, Apple are aggressive in getting the newest version of their OS onto people's phones as quickly as possible, and it's not like the days of Windows when a development tool could produce viable code for years and years and often there wasn't a compelling reason to keep upgrading. Someone has to do the work to keep Xamarin up to date with these mobile changes.
I personally love what they're trying to do, it's got me excited about development again after a few years of 'meh, it's just a day job', and I hope enough people can get past the pricing issue to see what you really get for your money. I appreciate that if you use VS too then it can get pricey, but VS isn't exactly cheap in itself. I looked into getting VS via an Azure and VS Online subscription and I think you can get VS Pro for about $40 a month that way, which would free-up short-term capital to get the more expensive Xamarin subscription.
Hey Stephan, can I assure you the answer to this question is yes, both to iOS and Android. I write both in objC and C#, and I can write two apps, one in Xcode, the other in Xamarin, and they will look no different and perform identically. Remember, monotouch is a library which binds the objc ios api to c#, there is no performance loss, (how can there be) there are many articles online which talk about this. The only difference is that your apps may be slightly larger. (and only by a couple of meg, as you need to export the binding libraries) Monodroid has actually been benchmarked to be faster then Dalvic on android, outperforming java.
Lots of whining about cost!
This isn't a platform for people who want to tinker and play. As AmbroseLittle mentioned, and I totally agree, some dev's expect everything for nothing, but love getting paid! Developing something as complex as Xamarin costs money, and Im not stupid enough to believe in the concept of a "free lunch". If your going to build an app, and you want it on iOS and Android, its really a no brainer. Html5 and Phonegap are great, as long as you only want to display webpages as apps, if you want serious native speed AND access to ALL the api for games (which is what I do) etc. then forget html5 for mobile, its just not up to the task...yet.
If your going to dev for iOS, its simple, BUY A MAC! You have no choice even if your on windows. (This obviously isn't Xamarins fault, blame Apple!) Xamarin studio on Mac is very similar to Visual Studio, they can even share and open sln files. I dev windows mobile on VisualStudio, in a VM inside my mac, and still share I would say 70% of code between all three, iOS, Win and Android. At 598 bucks a year for ios/droid indie ($11.50 per week) its well worth the time saving!
I'm not complaining about the pricing. It is what it is, and its out of reach for me at this point; I'm not the audience they're targeting. Which is unfortunate, because the system is bananas ( and bananas is good ). I'll likely move on to learning native development. Which in the long run is probably a more valuable endeavor for me anyway.
I'll definitely keep my eye on Xamarin though. They're doing a lot of really cool stuff, and I hope to be able to take advantage at some point.
Cheers
Alan.
Developers who are using free version of Xamarin will have to use Xamarin credited ads in their apps until it reaches amount of Xamarin wants (lets assume $299)
After application shows $299 valued ads credited to Xamarin they can take out the ad and replace with their own user control.
After do some research Apache Cordova it's enough to meat my requirement.
http://androidapi.xamarin.com/?link=N%3aAndroid.Hardware
Try navigating to the IFaceDetectionListener interface from:
http://androidapi.xamarin.com/?link=T%3aAndroid.Hardware.Camera%2bFace
"Error: Your request has found no candidate provider [hs="(null)", id="(null)"]" ?!
There's blank, placeholder or 404 stuff all over the place. It just doesn't instill confidence. (Mind you, MSDN docs seem to have gone downhill recently, too.) These were the first places I looked, btw - I hadn't been scouring the documentation looking for poor areas.
Likewise, having a free tier where you can't even compile an application that has a basic UI form or includes the portable HTTP library is pretty harsh. The other pricing plans are damned expensive, especially as they're annual subs.
Personally I believe the best option is the Xamarin route, Microsoft should buy them to bring the price down and at the same increase the apps for their own platform.
Other option as others have said is to have a valid indie developer license model, there is no need to explain that the adoption of developers of a platform will increase the number of licenses bought by current enterprises or future enterprises.
After all this years we might get the real (we all love) .net cross platform adoption which we talked 13? years ago. All the best to Miguel as I have followed the progress of Mono and other spin off projects / business and hopefully he makes it this time :)
I think you are all crazy ... it's very expensive!!!!!
A tool that helps us greatly increase productivity and costs a measly $24 a month "indie version", you spend it on dinners, outings or even beer with friends, all things uninteresting and you not complain. Actually I think $ 24 a month is quite reasonable for the tool in question. Rethink your priorities before you speak, even for students.
greetz
The price is an issue and I thing the $1000 for all platforms would be fairer and increase their customer base by more than double (therefore offsetting the price reduction).
The other issue I have is incorporating third party SDKs (e.g. facebook, adobe analytics, paypal).
You either have to create a C# binding project (which often isn't trivial) or hope that someone else has done it - and is keeping it up to date.
I personally would like to see Xamarin focussing their efforts on resolving this either by improving the tools to create the binding project, or preferably by maintaining all popular SDKs in their Components store.
Some truth about Xam's price:
1. If you want to use Visual Studio (required for Win Phone) then you're in the $999 pricing.
2. If you want to deploy to iOS then you must have access to a Mac, it's how debugging is possible -- for non-mac people like me that means purchasing a Mini.
3. It's not $999 per developer, it's $999 per developer PER PLATFORM (Mac, Android, Win) so that's up to $3000 for cross-platform deployment.
It's not the $999 price tag that is the problem, in fact that is probably fair. It's the ($999 + $999 + $999 + Mac Hardware) X DEVELOPER that is hard to swallow.
Considering the problem Xamarin is solving, honestly, they pretty much deserve a premium price. But, it won't be long before a competitor comes along and disrupts them... especially since the tooling is all open-standards so the switching cost will be relatively low.
well I'm not here to talk about that! I'm just curious to learn more about how they are using the MasterDetailPage control, specifically considering that they don't appear to be using XAML.
Is the XAML support less complete than the declarative model? Because every code sample I've seen is building the UI that way, while I, attempting to use XAML am having nothing but trouble.
For example, creating a plain-jane MasterDetailPage with static content (no binding of any kind, just a label) using XAML for each view, and run it, the view jumps straight to Detail view (I see the label for Details from that pane's content) and the content from the Master section is nowhere to be found.
How do I show the MasterPage by default? does it have to be bound to data?
I'm not looking for a specific answer to this as much as I'm trying to see if XAML is just a beta or what...
It really would be great to get more documentation on XAML from the Xamarin team; at this rate it appears that XAML may have just been an experiment and will probably not be supported fully for some time, am I wrong?
thanks
The engineering people at Xamarin have delivered something amazing i.e. take one of the world's most popular programming languages which is at the zenith of its adoption and allow it to be used to code, package and target the most popular devices for running program code.
Why then would Xamarin choose to price penalize its customers for trying to adopt Xamarin's unique selling point with a per mobile o/s cost? It must rank as the most absurd marking decision in the software industry today.
I am 3 weeks into my Xamarin trial and using Xamarin Studio. I laugh daily at the deficiencies of this free IDE because I know I will eventually be migrating to Visual Studio but any serious software team has to view $1000 per developer per target o/s as the minimum viable entry point, the $300 option is a nonsense option.
Developer sentiment and a groundswell of community adoption are vital in today's fashion conscious software tools landscape, for critical mass to be achieved. The pricing model is throttling this process and the pricing story has become the only Xamarin story in town which is drowning out the engineering story.
There also doesn't appear to be any discounts for annual renewals, which means you pony up the full price every year.
I also hope they look at other options to make it more viable to get started making money using VS. Perhaps limited support options (cutting down their support expenses), better discounts on combining platforms (paying for VS integration per platform seems rather steep), profit limitations before switching licenses etc.
Sinking this thread on their forum, http://forums.xamarin.com/discussion/9902/xamarin-license-is-not-perpetual-anymore, also gave me some concern about how the company handles customers. Note, they did try to enforce annual updates to be able to deploy and then reverted the decision, but tried to hide the thread.
I'm still looking at architecture and would have settled on Xamarin, but the price definitely is a strong factor and I keeps making me look for other options. I also came across Ionic/Cordova/AngularJs as interesting alternatives as someone else mentioned. As a C# developer, it is interesting times, do you continue with it, or move onto something else in the increasingly mobile world. Hopefully Xamarin can grow and get a larger user base and bring prices down. Good luck.
Curious for any feedback on this.
Comments are closed.