Easy accelerated 3D Games in a browser with JavaScript and WebGL using Three.js or Babylon.js
Let's ignore that we haven't got a web-agreed-upon way to do just about anything, much less basic line-of-business apps with data-bound text boxes over data, and just revel in the fact that we can do hardware-accelerated 3D graphics in our browsers with WebGL. This works today in all the latest browsers.
I noticed this fantastic mini-game promoting Assassin's Creed over at http://race.assassinscreedpirates.com that uses WebGL via a library called Babylon.js. On my Windows machine it runs perfectly in IE11, Chrome 37, or Firefox 30. (Yes, in retrospec, the version numbering for browsers is getting out of hand.)
Some folks on Macs have reported issues with WebGL. Check out https://www.browserleaks.com/webgl for YOUR browser's capabilities.
This Pirate Ship game is really worth checking out and it's fun for the kids. You can also check out the Developer Teardown and learn how they built it.
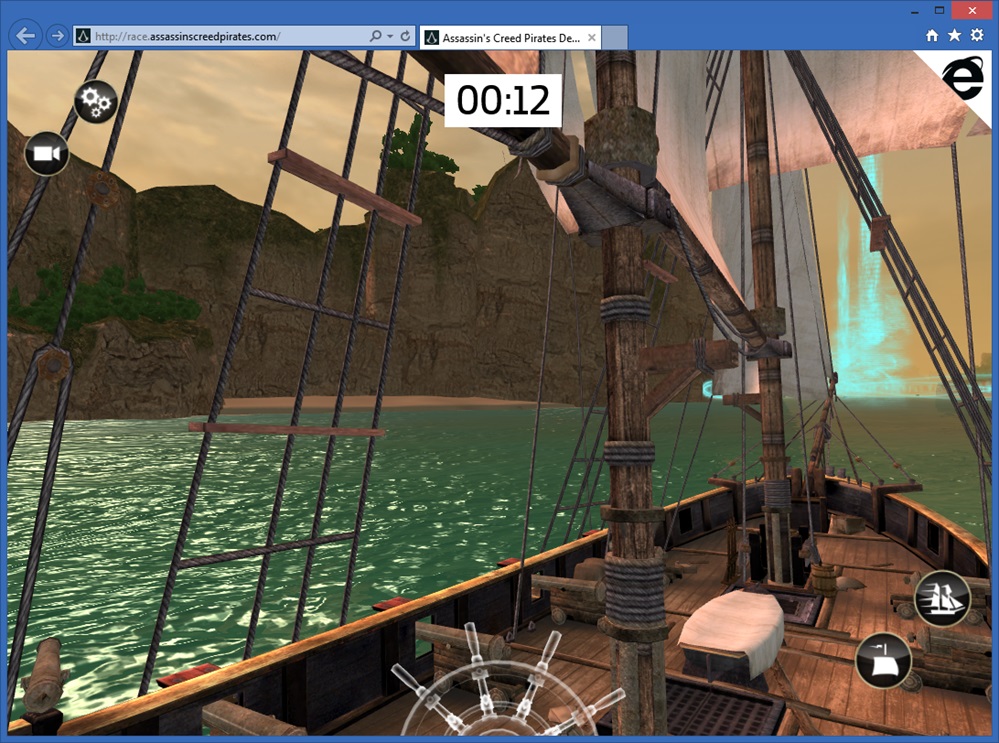
I wanted to get a better understanding of exactly how difficult it is (or easy!) to get in to WebGL in the browser. I found this article over at SitePoint by Joe Hewitson and started with some of his sample code where he compares two popular WebGL libraryes, three.js and babylon.js. The Assassin's Creed game here is written using Babylon.js.
Three.js seem to be a general layer on top of WebJS, aiming to make scene creation and animation easy. Using Joe's sample (that I changed a little) along with a texture.gif, I was able to make this spinning 3D cube easily.
There's a live example running at http://hanselstorage.blob.core.windows.net/blog/WebGL.html you can see in your modern browser. Below is just a static image.
Three.js is on the left and Babylon.js is on the right. It doesn't really matter given the static image, but one think you'll notice immediately if you run the live sample is that you can zoom in and out, pan, grab, and manipulate the babylon.js cube. I could have hooked up some events to the three.js cube, or perhaps added a physics engine like Physijs and made it interact with the world, I suppose. It struck me though, that this little example shows the difference in philosophy between the two. Babylon seems to be more of a game engine or a library that wants to help you make games so there's interactions, collision detection, and lighting included.
Three.js supports many renderers, cameras, and lighting. You can use all WebGL capabilities such as lens flares. It supports all the usual objects and geometries as well. Their examples are extensive with over 150 coding examples covering everything you'd want to know from fonts, models, textures, to sounds. There's even an in-browser Three.js editor.
Babylon includes a lot including a physics engine from cannon.js built in, full scene graph, offline mode, textures, special effects and post processes, many cameras including Oculus Rift (!) lots of meshes. Babylon.js even supports standard Gamepads via like the Xbox One controller natively via the Gamepad API spec.
Anyway, here is the cube on the right from Joe's code that I modified slightly, using babylon.js:
// Babylon.js
var canvas = document.getElementById('babylonCanvas');
var engine = new BABYLON.Engine(canvas, true);
var sceneB = new BABYLON.Scene(engine);
var camera = new BABYLON.ArcRotateCamera("camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), sceneB);
var light = new BABYLON.DirectionalLight("light", new BABYLON.Vector3(0, -1, 0), sceneB);
//light.diffuse = new BABYLON.Color3(1, 0, 0);
//light.specular = new BABYLON.Color3(1, 1, 1);
var box = BABYLON.Mesh.CreateBox("box", 3.0, sceneB);
var material = new BABYLON.StandardMaterial("texture", sceneB);
box.material = material;
material.diffuseTexture = new BABYLON.Texture("texture.gif", sceneB);
sceneB.activeCamera.attachControl(canvas);
engine.runRenderLoop(function () {
box.rotation.x += 0.005;
box.rotation.y += 0.01;
sceneB.render();
});
I also commented out the lighting but you can see how easy it is to add lighting to a scene if you like. In this case there was a diffuse red light along with a specular white.
With babylon.js I could change the size of the scene by changing the size of the canvas. With three.js the width and height are pulled in programmatically from the enclosing div.
Here is the near-same box created with three.js.
// Three.js
var div = document.getElementById('three');
var height = div.offsetHeight;
var width = div.offsetWidth;
var renderer = new THREE.WebGLRenderer();
renderer.setSize(width, height);
div.appendChild(renderer.domElement);
var camera = new THREE.PerspectiveCamera(70, width / height, 1, 1000);
camera.position.z = 400;
var sceneT = new THREE.Scene();
var cube = new THREE.CubeGeometry(200, 200, 200);
var texture = THREE.ImageUtils.loadTexture('texture.gif');
texture.anisotropy = renderer.getMaxAnisotropy();
var material = new THREE.MeshBasicMaterial({ map: texture });
var mesh = new THREE.Mesh(cube, material);
sceneT.add(mesh);
animate();
function animate() {
requestAnimationFrame(animate);
mesh.rotation.x += 0.005;
mesh.rotation.y += 0.01;
renderer.render(sceneT, camera);
}
Both libraries have amazing Examples Galleries and you should check them out.
Also note that there's a contest running until June 20th, 2014 over at http://www.modern.ie/en-us/demos/assassinscreedpirates where you'll create your own shader within the browser and submit it for judging. Contestants must be over 16 years of age and from one of the following countries: United States of America, United Kingdom, France, Germany, Spain, or Australia. The contest starts here and the grand prize is an Assassin’s Creed Collector’s Black Chest Edition and an Xbox One.
Even if you don't win, do check out the in-browser shader editor. It's amazing.
Sponsor: A big welcome to my friends at Octopus Deploy. They are sponsoring the blog feed this week. Using NuGet and powerful conventions, Octopus Deploy makes it easy to automate releases of ASP.NET applications and Windows Services. Say goodbye to remote desktop and start automating today!
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
I've also done a 1 hour video overview of Babylon.js on YouTube: https://www.youtube.com/watch?v=z80TYMqsdEM
Regards.
You'll need to do ...
var gl =canvas.getContext("experimental-webgl");
But apart from that, it seems like a working (though presumably partial) WebGL on there.
Tizen 2 is Webkit based. For 3 they are switching to Crosswalk.
Have fun!
Comments are closed.