Exploring a minimal WebAPI with .NET Core and NancyFX
In my last blog post I was exploring a minimal WebAPI with ASP.NET Core. In this one I wanted to look at how NancyFX does it. Nancy is an open source framework that takes some inspiration from Ruby's "Sinatra" framework (get it? Nancy Sinatra) and it's a great alternative to ASP.NET. It is an opinionated framework - and that's good thing. Nancy promotes what they call the "super-duper-happy-path." That means things should just work, they should be easy to customize, your code should be simple and Nancy itself shouldn't get in your way.
As I said, Nancy is open source and hosted on GitHub, so the code is here https://github.com/NancyFx/Nancy. They're working on a .NET Core version right now that is Nancy 2.0, but Nancy 1.x has worked great - and continues to - on .NET 4.6 on Windows. It's important to note that Nancy 1.4.3 is NOT beta and it IS in production.
As of a few weeks ago there was a Beta of Nancy 2.0 on NuGet so I figured I'd do a little Hello Worlding and a Web API with Nancy on .NET Core. You should explore their samples in depth as they are not just more likely to be correct than my blog, they are just MORE.
I wanted to host Nancy with the ASP.NET Core "Kestrel" web server. The project.json is simple, asking for just Kestrel, Nancy, and the Owin adapter for ASP.NET Core.
{ "version": "1.0.0-*", "buildOptions": { "debugType": "portable", "emitEntryPoint": true }, "dependencies": { "Microsoft.NETCore.App": { "version": "1.0.0", "type": "platform" }, "Microsoft.AspNetCore.Server.Kestrel": "1.0.0", "Microsoft.AspNetCore.Owin": "1.0.0", "Nancy": "2.0.0-barneyrubble" }, "commands": { "web": "Microsoft.AspNet.Server.Kestrel" }, "frameworks": { "netcoreapp1.0": {} } }
And the Main is standard ASP.NET Core preparation. setting up the WebHost and running it with Kestrel:
using System.IO;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
namespace NancyApplication
{
public class Program
{
public static void Main(string[] args)
{
var host = new WebHostBuilder()
.UseContentRoot(Directory.GetCurrentDirectory())
.UseKestrel()
.UseStartup<Startup>()
.Build();
host.Run();
}
}
}
Startup tells ASP.NET Core via Owin that Nancy is in charge (and sure, didn't need to be its own file or it could have been in UseStartup in Main as a lambda)
using Microsoft.AspNetCore.Builder; using Nancy.Owin; namespace NancyApplication { public class Startup { public void Configure(IApplicationBuilder app) { app.UseOwin(x => x.UseNancy()); } } }
Here's where the fun stuff happens. Check out a simple Nancy Module.
using Nancy; namespace NancyApplication { public class HomeModule : NancyModule { public HomeModule() { Get("/", args => "Hello World, it's Nancy on .NET Core"); } } }
Then it's just "dotnet restore" and "dotnet run" and I'm in business. But let's do a little more. This little bit was largely stolen from Nancy's excellent samples repository. Here we've got another route that will respond to a GET to /test/Scott, then make and return a new Person() object. Since I'm going to pass in the Accept: application/json header I'll get JSON back.
using Nancy; namespace NancyApplication { public class HomeModule : NancyModule { public HomeModule() { Get("/", args => "Hello from Nancy running on CoreCLR"); Get("/test/{name}", args => new Person() { Name = args.name }); } } public class Person { public string Name { get; set; } } }
I'm using the Postman application to test this little Web API and you can see the JSON response below:
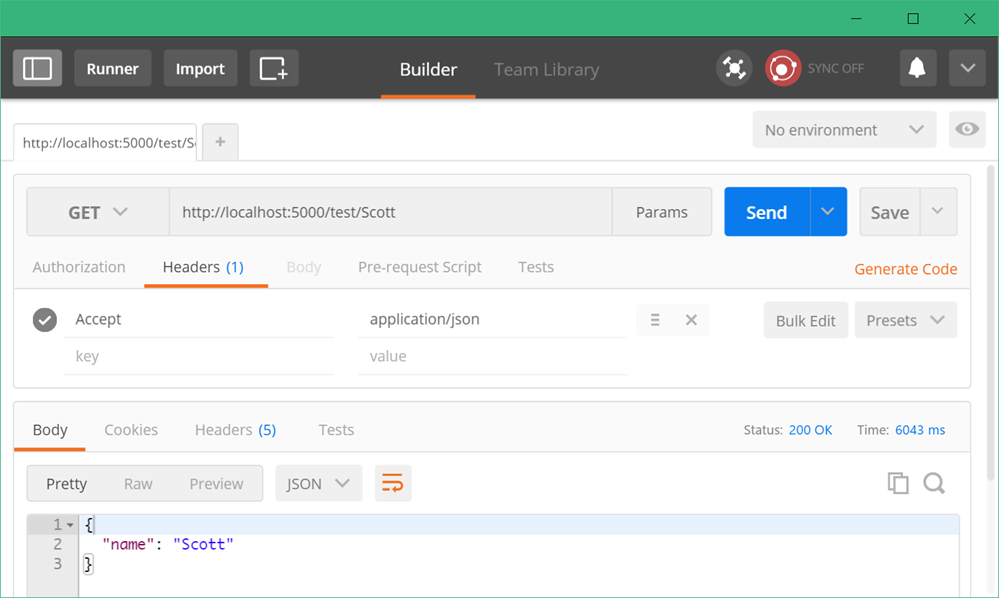
Nancy is a very complete and sophisticated framework with a LOT of great code to explore. It's extremely modular and flexible. It works with ASP.NET, with WCF, on Azure, with Owin, alongside Umbraco, with Mono, and so much more. I'm looking forward to exploring their .NET Core version as it continues development.
Finally, if you're a new open source contributor or are considering being a First Timer and help out an open source project, you might find the idea of getting involved with such a sophisticated project intimidating. Nancy participates in UpForGrabs and has some issues that are marked as "up for grabs" that could be a good starter point where you could help out a deserving project AND get involved in open source.
* WoCTechChat photo used under CC
Sponsor: Thanks to Aspose for sponsoring the feed this week! Aspose makes programming APIs for working with files, like: DOC, XLS, PPT, PDF and countless more. Developers can use their products to create, convert, modify, or manage files in almost any way. Aspose is a good company and they offer solid products. Check them out, and download a free evaluation!
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
Thanks for the article! :-)
I just wonder if WebAPI is going to have an upgraded version for ASP.NET Core, something like WebApi 3.0?
Cheers, Sia
I suggest you read Scott's previous post. It explains that in .NET Core view and api controllers use the same base class. In other words webapi 3.0 is already in .net core.
Note this package.json won't work with dotnet.exe 1.0.0-preview1-002702. So update your dotnet.exe to avoid headaches.
Yeoman ASPNet Generator link here for others
I have been a NancyFx user for almost 3 years now. We have a complex set of REST services built on top of Nancy with custom security and cache loading incorporated into the startup and Before pipelines in Nancy. Nancy is by far the best low ceremony web framework I have used!
Thanks for spotlighting this great framework for the .NET community!
Shane
I am user of NancyFx for almost 2 years . We have a complex set of REST services built on top of Nancy with custom security and cache loading incorporated into the startup and Before pipelines in Nancy.
best ed treatment
Comments are closed.
If anyone has any questions about Nancy feel free to jump onto our Slack channel http://slack.nancyfx.org/, raise a Github issue or ping us @NancyFX on twitter.
We'd love more people testing our v2 (now on .Net Core) and more people submitting PRs and contributing to Nancy.
I started with Nancy in 2012, not knowing Git and was helped out by the Nancy team to learn it. We try to be as helpful and accommodating to seasoned pros and beginners.
Come and try Nancy and see all the fun you're missing =)
Jonathan