One ASP.NET: Nancy.Templates for Visual Studio
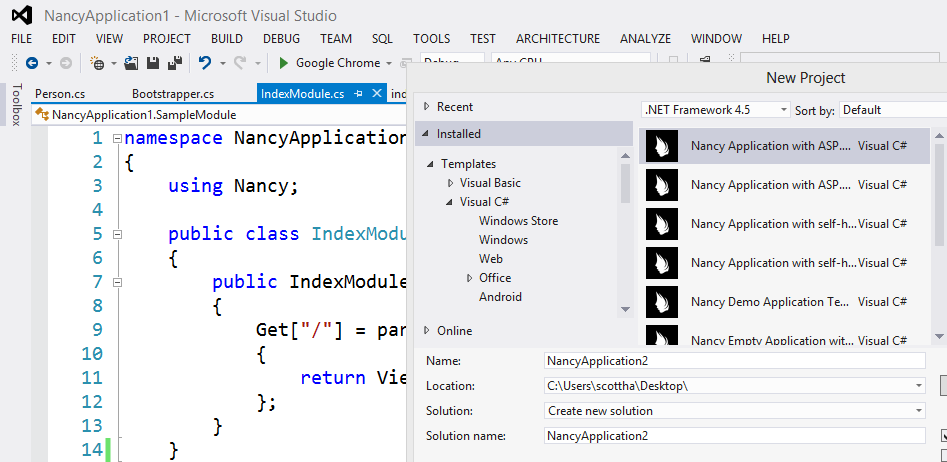
I hope you've updated to Visual Studio 2012.2 and picked up Web Essentials because we're continuing to add goodness all the time. As we march forward with the One ASP.NET vision, so does the community. One of the major goals has been to make it easier for the community to not only make templates but also live alongside ASP.NET templates as peers. This has been historically hard. It's still too complex, in fact, but it's easier than before. I'm hoping that one day soon making templates will be as easy as making and sharing NuGet packages.
Not only is most of ASP.NET open source, but so are the Azure SDKs, NuGet and more. However, there's a large and rich world of open source frameworks and projects that some companies never get to use either because their company isn't into Open Source or because they won't use code that doesn't come from Microsoft.
Additionally, Microsoft, IMHO, has done a poor job (as a collective) letting developers know that there are options and options are good. Personally, I don't care if you use Entity Framework, or Web Forms, or MVC or Web API. You can use NHibernate, Nancy, Simple.Web, ServiceStack and OpenRasta. It makes no difference to me or my organization. If you are happy and using .NET, then I'm happy and that's great. Microsoft want you to use Azure and Windows, I'm sure, but after that ultimately the rest is just the details of your stack. You should explore the options available and work within your organization to be successful.
On the topic of options, NancyFx is an open source web framework for .NET that uses the Ruby Framework "Sinatra" as its inspiration. (Get it? Frank Sinatra's daughter is Nancy Sinatra.)
A few days ago (with some gentle prodding, and some great team effort) the NancyFx team created a VSIX to integrate the NancyFx Web Framework into the Visual Studio project dialog. (You can also get NancyFx from NuGet, of course.)
It's totally simple from your point of view, as it should be. Download the VSIX, and double click on it. Done.
Now, in Visual Studio, just File | New Project and you've got more choices!
Nancy is a very lightweight and flexible framework for web sites and services. You don't even need ASP.NET proper. You can self-host in your own services or exe, or host within ASP.NET. You can use Razor syntax or choose other View Engines.
Here's Hello World:
public class SampleModule : Nancy.NancyModule
{
public SampleModule()
{
Get["/"] = _ => "Hello World!";
}
}
Interesting and very concise convention, eh? The Get["/"] syntax is a route, saying that an HTTP GET for / should be handled by this => anonymous method. It's all C#, mind you!
Here's the next step, passing a model into a View:
public class SampleModule : NancyModule
{
public SampleModule()
{
Get["/"] = parameters => {
var person = new Person {
Id = 1,
Name = "Scott Hanselman",
Content = "Lorem ipsum...",
Tags = {"c#", "aspnet", "oss", "nancy"}
;
return View["Index", person];
};
}
}
Nice, eh? Very familiar if you're comfortable with ASP.NET MVC and general MVC-style frameworks.
If you want to return Json, you can take an object and call AsJson() like this:
Get["/person"] = parameters =>
{
var person = new
{
Id = 1,
Name = "Scott Hanselman",
Content = "Lorem ipsum..."
};
return Response.AsJson(person);
};
UPDATE: I am reminded by commenters that Nancy now supports Content Negotiation ("conneg") and will automatically return the format that the client requested. This means if your HTTP headers say "accept: application/json" then you'll get JSON back automatically. Lovely, and no need for the AsJson() (unless that's what you wanted).
There's samples you can see at http://samples.nancyfx.org and you are encouraged to add your own sample. Just File | New Project, and make a Nancy Demo Application and follow the instructions.
NancyFx has a great community of users and you'll often find them chatting on Jabbr or in their Google Group. There's even a Resharper Plugin for Nancy. Nancy also runs nicely on Mac with Mono and MonoDevelop.
Kudos and congrats to the Nancy team for being awesome, and for making these templates.
Related Links
SPONSOR: Big thanks to the feed sponsor this week, Ext.NET (seriously, check out their demos, really amazing stuff!) - Quickly build modern WebForm and MVC (including RAZOR) Apps for ASP.NET. Free pancake breakfast with all purchases!
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
Cya in there!
http://www.philliphaydon.com/2013/04/nancyfx-revisiting-content-negotiation-and-apis-part-1
Can read this to find out more about conneg with NancyFX.
I really like the idea of having multiple choices for different circumstances, and I do like some of the ideas provided by NancyFx. But what I do not like is the use of literals throughout the code.
The great thing about MVC is, by default each action name corresponds to a path, like public ActionResult Persons() can be called using /controller/Persons.
If you want to change the route, it only has to be in one place. I see some big problems ahead using NancyFX if you want to use other route names or otherwise want to refactor your code. You can't just rename a string or a dynamic property if you use the same property multiple times.
In my opinion, NancyFX is not mature enough to replace MVC
The default routing forces you into a particular design of routes/naming, and the moment you need to do something different you need to create a custom route definition, which is hidden away, and non-predictable. The default implementation is also not as flexible as Nancy, again requiring AR to solve a lot of those problems.
Also its not really fair to say its not mature because of literals. Theres plenty of production deployments of large applications using Nancy that get along just fine. A well designed API prevents you worring about things like renaming an action.
I don't want to argue, just my opinion in response to your opinion :)
Nancy is mature enough to make complete enterprise level application. It is just how you want to proceed further. Like, if I start working on something and if I process requirement in GET, POST, PUT, DELETE in REST fashion I will select Servicestack or Web api. Personally I go with Servicestack, just personal choice. But if I feel like request and pages then NancyFX. If I am working in team and I have time and resource to boot the project and wanted to working in convention based environment will go for Asp.Net MVC or Fubu MVC. It just a choice nothing else. Even I give equal preference for C# and F#. As normally Scott use to tell, use what you fill using. Its just like that. For further clarification this article may help http://odetocode.com/blogs/scott/archive/2013/02/12/you-want-to-build-web-software-with-c.aspx
@scott Thanks for great article.
I tried creating 2 web applications. The first one is using Nancy and the second one is an empty MVC4 application. In empty MVC4 applications I added Home Controller and Index View which contains "Index" text only. On Nancy web application the Index view contains also "Index" text only. Running the two applications I observed the rendered time using Firebug. I found out that Nancy Index page is loaded as fast as 14ms while MVC4 Index is loaded as fast as 4ms. I keep on trying thinking that it happened only by chance but no luck. MVC4 is loading faster than Nancy.
My conclusion is Nancy CANNOT offer better performance compare to MVC Framewowrk base on my bench mark.
Your firebug test is meaningless.
http://i.imgur.com/n0obYyS.png
Also NancyFX has 0 dependency on System.Web, so yes its very lightweight.
I also recently also found out about Django support in Visual Studio 2012.
If you are interested in more Microsoft-Open-Source goodness then checkout http://www.microsoft.com/en-us/openness/default.aspx#projects
I've googled and looked around the Nancy web site but don't see the VS addin.
Thanks, Scott
https://github.com/NancyFx/Nancy.Templates
Comments are closed.
Me too! Instead of making them "as easy as" NuGet packages, let's actually use NuGet packages themselves for this. While we're at it, let's make it so you can easily mix and match them (ie create a Nancy project using Entity Framework, Autofac, and MSTest), and go back to the "Project creation" dialog and change your choices after you've already created the project.
I proposed this a bit ago on the ASP.NET forms: Flexible Project Templates