Peachpie - Open Source PHP Compiler to .NET and WordPress under ASP.NET Core
The Peachpie PHP compiler project joined the .NET Foundation this week and I'm trying to get my head around it. PHP in .NET? PHP on .NET? Under .NET? What compiles to what? Why would I want this? How does it work? Does it feel awesome or does it feel gross?
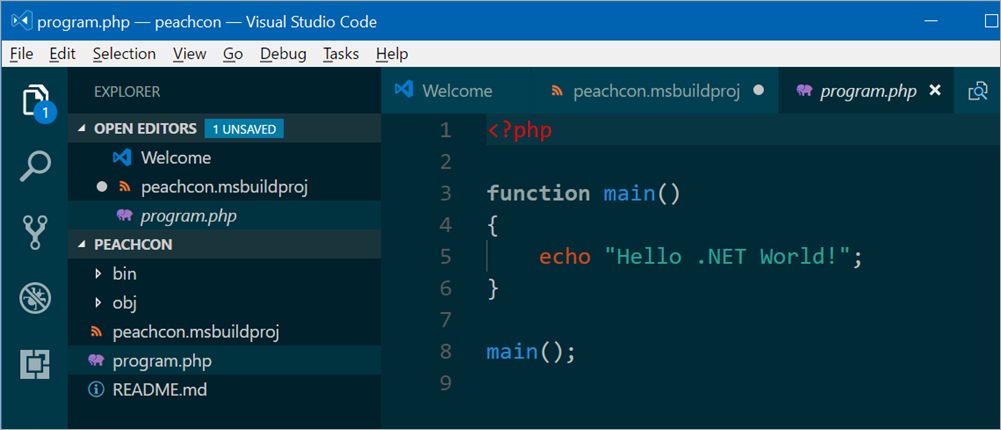
Just drink this in.
C:\Users\scott\Desktop\peachcon> type program.php
<?php
function main()
{
echo "Hello .NET World!";
}
main();
C:\Users\scott\Desktop\peachcon> dotnet run
Hello .NET World!
Just like that. Starting from a .NET SDK (They say 1.1, although I used a 2.0 preview) you just add their templates
dotnet new -i Peachpie.Templates::*
Then dotnet new now shows a bunch of php options.
C:\Users\scott\Desktop\peachcon> dotnet new | find /i "php"
Peachpie console application peachpie-console PHP Console
Peachpie Class library peachpie-classlibrary PHP Library
Peachpie web application peachpie-web PHP Web/Empty
dotnet new peachpie-console for example, then dotnet restore and dotnet run. Boom.
NOTE: I did have to comment out his one line "<Import Project="$(CSharpDesignTimeTargetsPath)" />" in their project file that doesn't work at the command line. It's some hack they did to make things work in Visual Studio but I'm using VS Code. I'm sure it's an alpha-point-in-time thing.
It's really compiling PHP into .NET Intermediate Language!
You can see my string here:
But...why? Here's what they say, and much of it makes sense to me.
- Performance: compiled code is fast and also optimized by the .NET Just-in-Time Compiler for your actual system. Additionally, the .NET performance profiler may be used to resolve bottlenecks.
- C# Extensibility: plugin functionality can be implemented in a separate C# project and/or PHP plugins may use .NET libraries.
- Sourceless distribution: after the compilation, most of the source files are not needed.
- Power of .NET: Peachpie allows the compiled WordPress clone to run in a .NET JIT'ted, secure and manageable environment, updated through windows update.
- No need to install PHP: Peachpie is a modern compiler platform and runtime distributed as a dependency to your .NET project. It is downloaded automatically on demand as a NuGet package or it can be even deployed standalone together with the compiled application as its library dependency.
PHP does have other VMs/Runtimes that are used (beyond just PHP.exe) but the idea that I could reuse code between PHP and C# is attractive, not to mention the "PHP as dependency" part. Imagine if I have an existing .NET shop or project and now I want to integrate something like WordPress?
PHP under ASP.NET Core
Their Web Sample is even MORE interesting, as they've implemented PHP as ASP.NET Middleware. Check this out. See where they pass in the PHP app as an assembly they compiled?
using Peachpie.Web;
namespace peachweb.Server
{
class Program
{
static void Main(string[] args)
{
var host = new WebHostBuilder()
.UseKestrel()
.UseUrls("http://*:5004/")
.UseStartup<Startup>()
.Build();
host.Run();
}
}
class Startup
{
public void ConfigureServices(IServiceCollection services)
{
// Adds a default in-memory implementation of IDistributedCache.
services.AddDistributedMemoryCache();
services.AddSession(options =>
{
options.IdleTimeout = TimeSpan.FromMinutes(30);
options.CookieHttpOnly = true;
});
}
public void Configure(IApplicationBuilder app)
{
app.UseSession();
app.UsePhp(new PhpRequestOptions(scriptAssemblyName: "peachweb"));
app.UseDefaultFiles();
app.UseStaticFiles();
}
}
}
Interesting, but it's still Hello World. Let's run WordPress under PeachPie (and hence, under .NET). I'll run MySQL in a local Docker container for simplicity:
docker run -e MYSQL_ROOT_PASSWORD=password -e MYSQL_DATABASE=wordpress -p 3306:3306 -d mysql
I downloaded WordPress from here (note they have the "app" bootstrapper" that hosts .NET and then runs WordPress) restore and run.
It's early and it's alpha - so set your expectations appropriately - but it's surprisingly useful and appears to be under active development.
What do you think?
Be sure to explore their resources at http://www.peachpie.io/resources and watch their video of WordPress running on .NET. It's all Open Source, in the .NET Foundation, and the code is up at https://github.com/iolevel/ and you can get started here: http://www.peachpie.io/getstarted
Sponsor: Check out JetBrains Rider: a new cross-platform .NET IDE. Edit, refactor, test and debug ASP.NET, .NET Framework, .NET Core, Xamarin or Unity applications. Learn more and download a 30-day trial!
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
In my opinion, language interop between runtimes is more maintainable and leaner than re-implementation of the existing language on a completely different runtime. There will always be differences with the main implementation and incompatibility with existing libraries due to the dependence on runtime features such as GC, call stack, exceptions, etc.
But this is a nice marketing for PHP developers to get introduced into .NET ;)
Maybe image processing, audio file metadata handling, video file metadata handling, ...
How's this different than IronPython and IronRuby which lasted 4 years (2006 - 2010) before being abandoned by MS?
More information here: Exotic PHP implementations: HippyVM, JPHP, Tagua VM, Peachpie
Does it support version 2.0.0-preview2-006497 ?
I tried the following:
dotnet new -i Peachpie.Templates::*
dotnet new peachpie-console
Then when I ran dotnet restore, I got the following message:
c:\FirstPeachpie\FirstPeachpie.msbuildproj(22,3): error MSB4020: The value "" of the "Project" attribute in element <Import> is invalid.
I don't know if it has something to do with the dotnet version I'm using. But when I tried the same steps above with version 1.1, it worked fine.
Comments are closed.
So, a way to run a php backend with more horsepower?