WinAppDriver - Test any app with Appium's Selenium-like tests on Windows
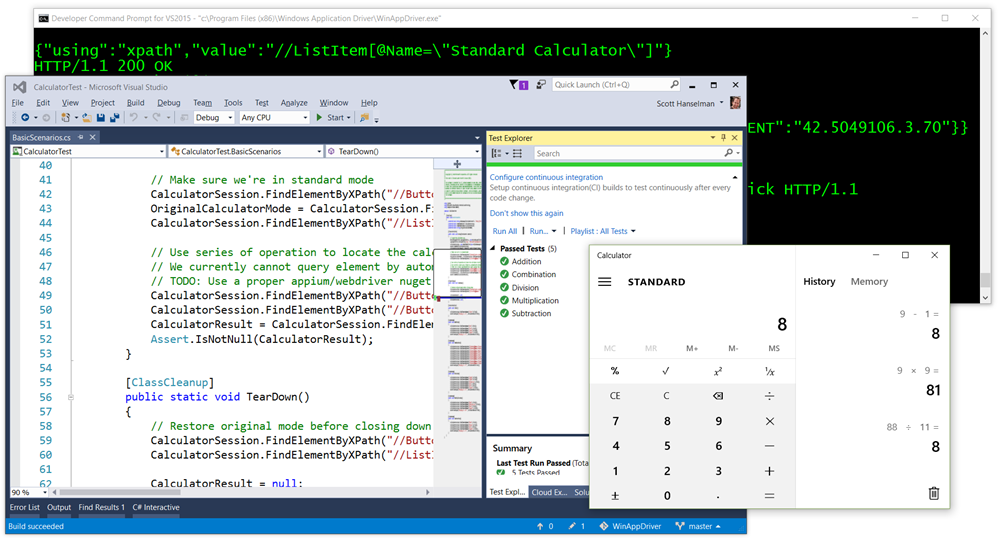
I've found blog posts on my site where I'm using the Selenium Web Testing Framework as far back as 2007! Today there's Selenium Drivers for every web browser including Microsoft Edge. You can write Selenium tests in nearly any language these days including Ruby, Python, Java, and C#.
I'm a big Selenium fan. I like using it with systems like BrowserStack to automate across many different browser on many operating systems.
"Appium" is a great Selenium-like testing framework that implements the "WebDriver" protocol - formerly JsonWireProtocol.
WebDriver is a remote control interface that enables introspection and control of user agents. It provides a platform- and language-neutral wire protocol as a way for out-of-process programs to remotely instruct the behavior of web browsers.
From the Appium website, "Appium is 'cross-platform': it allows you to write tests against multiple platforms (iOS, Android, Windows), using the same API. This enables code reuse between iOS, Android, and Windows testsuites"
Appium is a webserver that exposes a REST API. The WinAppDriver enables Appium by using new APIs that were added in Windows 10 Anniversary Edition that allow you to test any Windows app. That means ANY Windows App. Win32, VB6, WPF, UWP, anything. Not only can you put any app in the Windows Store, you can do full and complete UI testing of those apps with a tool that is already familiar to Web Developers like myself.
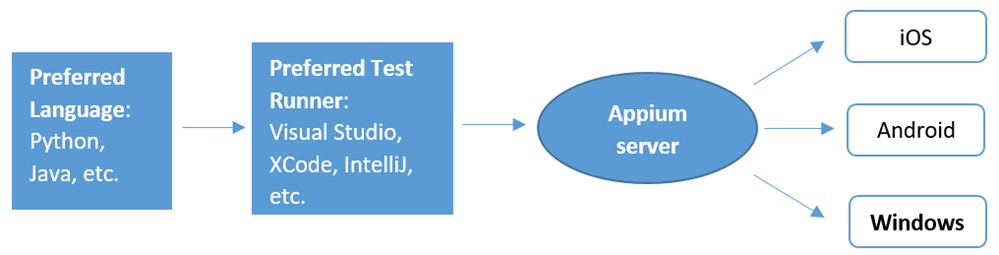
You can write tests in C# and run them from Visual Studio's Test Runner. You can press any button and basically totally control your apps.
// Launch the calculator app
DesiredCapabilities appCapabilities = new DesiredCapabilities();
appCapabilities.SetCapability("app", "Microsoft.WindowsCalculator_8wekyb3d8bbwe!App");
CalculatorSession = new RemoteWebDriver(new Uri(WindowsApplicationDriverUrl), appCapabilities);
Assert.IsNotNull(CalculatorSession);
CalculatorSession.Manage().Timeouts().ImplicitlyWait(TimeSpan.FromSeconds(2));
// Make sure we're in standard mode
CalculatorSession.FindElementByXPath("//Button[starts-with(@Name, \"Menu\")]").Click();
OriginalCalculatorMode = CalculatorSession.FindElementByXPath("//List[@AutomationId=\"FlyoutNav\"]//ListItem[@IsSelected=\"True\"]").Text;
CalculatorSession.FindElementByXPath("//ListItem[@Name=\"Standard Calculator\"]").Click();
It's surprisingly easy once you get started.
public void Addition()
{
CalculatorSession.FindElementByName("One").Click();
CalculatorSession.FindElementByName("Plus").Click();
CalculatorSession.FindElementByName("Seven").Click();
CalculatorSession.FindElementByName("Equals").Click();
Assert.AreEqual("Display is 8 ", CalculatorResult.Text);
}
You can automate any part of Windows, even the Start Menu or Cortana.
var searchBox = CortanaSession.FindElementByAccessibilityId("SearchTextBox");
Assert.IsNotNull(searchBox);
searchBox.SendKeys("What is eight times eleven");
var bingPane = CortanaSession.FindElementByName("Bing");
Assert.IsNotNull(bingPane);
var bingResult = bingPane.FindElementByName("88");
Assert.IsNotNull(bingResult);
If you use "AccessibiltyIds" and refer to native controls in a non-locale specific way you can even reuse test code across platforms. For example, you could write sign in code for Windows, iOS, your web app, and even a VB6 Win32 app. ;)
Appium and WebAppDriver a nice alternative to "CodedUI Tests." CodedUI tests are great but just for Windows apps. If you're a web developer or you are writing cross platform or mobile apps you should check it out.
- WinAppDriver Issues & Samples
- Learn more about Appium
- UI Test Automation for Browsers and Apps Using the WebDriver Standard
Sponsor: Help your team write better, shareable SQL faster! Discover how your whole team can write better, shareable SQL faster with a free trial of SQL Prompt. Write, refactor and share SQL effortlessly, try it now.
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter
But I wanted to bring to your notice a development that will affect us. Visual Studio Community Edition moved from "for small professional teams" to strictly "individuals" or open-source. Why you do this?
The terms linked in the VS 2017 Community RC installer seem to be the same as VS 2015 Community with regards to how it can be used for individuals and organizations - Here
Admittedly I only scanned through the pages linked to and the examples they showed (didn't download anything) but I couldn't seem to find anything that tells you how you find out the names of stuff, e.g.:
appCapabilities.SetCapability("app", "Microsoft.WindowsCalculator_8wekyb3d8bbwe!App");
Where do you find the 2nd parameter value?
Or when you mention automating Windows:
var searchBox = CortanaSession.FindElementByAccessibilityId("SearchTextBox");
How would someone without access to the source code find out the value "SearchTextBox", assuming that is what it's called in the source code.
If you had a WinForms app would the name be the same as the name in the code, e.g. if I had a checkbox called doYouWantToSuperSizeThatCheckBox would I simply use that name in my tests?
In the case of .NET what about if you've obfuscated the assembly?
What are "new APIs that were added in Windows 10 Anniversary Edition that allow you to test any Windows app" ?
Where are those listed ?
Thank you
Andrei
The terms linked in the VS 2017 Community RC installer seem to be the same as VS 2015 Community with regards to how it can be used for individuals and organizations
Thanks Anonymous Coward. Marketing material suggests otherwise. I guess we will have to wait for RTM so that there is clarity on this.
C:\Program Files (x86)\Windows Kits\10\bin\x86\inspect.exe
Thanks.
Here's the WPF bug: https://github.com/Microsoft/WinAppDriver/issues/83
We'll continue adding samples to showcase the various types of apps you can test. If you run into other issues please file them on the GitHub project's issue tracking and we'll take a look.
@JG thanks for calling out the inspect tool. Agree inspect is a very useful tool.
Yosef
@yosefdurr
https://channel9.msdn.com/Events/Connect/2016/202
Comments are closed.
What versions of the tools\OS are required for this to work?
http://i.imgur.com/NuHeweI.png